What is javascript? Everything you need to know
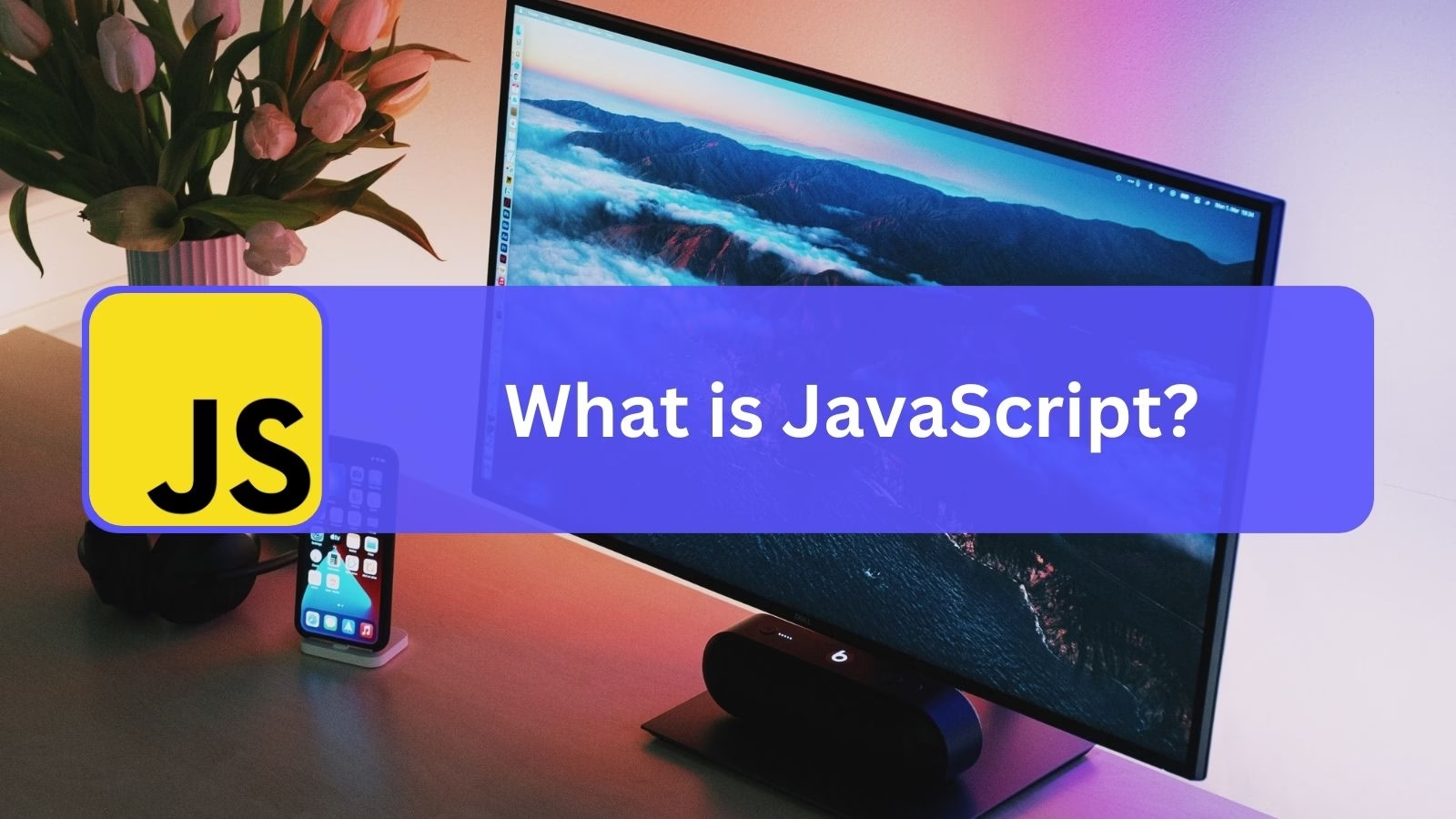
This post was generated with AI and then edited by us.
JavaScript is a high-level, dynamic programming language that plays a vital role in web development. Initially created in 1995 by Brendan Eich while he was at Netscape, JavaScript was designed to add interactivity to web pages. At that time, the language was referred to as Mocha, and later renamed to LiveScript before finally adopting its current name, JavaScript. This evolution in naming reflects a strategic move to capitalize on the growing reputation of Java, which was gaining popularity as a robust programming language.
What is JavaScript and Why It’s So Popular
JavaScript is a high-level programming language that adds interactivity and dynamic features to websites, such as form validation, animations, and real-time content updates. It runs on the client-side (the user’s device) rather than the server, which improves performance and reduces server load. Paired with HTML for structure and CSS for styling, JavaScript enables developers to create rich and responsive web experiences.
JavaScript’s popularity stems from its versatility. It’s used for both front-end and back-end development with tools like Node.js, making it easier to build full-stack applications with one language. It’s also supported across all major browsers, ensuring broad compatibility. The language’s easy syntax, vast ecosystem of frameworks like React and Angular, and an active developer community have further fueled its rise.
JavaScript enables cross-platform development for mobile and desktop apps through tools like React Native and Electron. This combination of flexibility, accessibility, and strong community support has made JavaScript the go-to language for modern web development.
JavaScript execution – How does JavaScript work?
JavaScript is executed by specialized programs called JavaScript engines, which are embedded in browsers (e.g., Chrome, Firefox, Safari) or runtime environments like Node.js. The process can be divided into two perspectives: browser-specific workflow (loading, parsing, and executing JavaScript for web pages) and engine internals (handling, optimizing, and executing JavaScript code). Here’s a unified explanation:
1. JavaScript Execution in the Browser
When you open a web page:
- HTML and CSS Loading:
- The browser fetches and parses HTML and CSS to build:
- The DOM (Document Object Model), representing the structure of the page.
- The CSSOM (CSS Object Model), representing the styles.
- These are combined to render the visual layout of the page.
- The browser fetches and parses HTML and CSS to build:
- JavaScript Loading:
- The browser identifies
<script>
tags and downloads the associated JavaScript code (either inline or in external.js
files). - By default, scripts block rendering while they execute unless they include the
async
ordefer
attributes.
- The browser identifies
- Integration with the DOM and CSSOM:
- JavaScript can manipulate the DOM and CSSOM to dynamically change the structure or appearance of the page (e.g., updating text, modifying styles, or adding elements).
2. How the JavaScript Engine Works
Every browser has a built-in JavaScript engine, such as:
- V8 (Chrome, Edge, Node.js)
- SpiderMonkey (Firefox)
- JavaScriptCore (Safari)
Here’s how the engine processes and executes JavaScript:
- Parsing
- The engine reads the source code and converts it into an Abstract Syntax Tree (AST) through two stages:
- Lexical Analysis: The code is broken into tokens (e.g.,
function
,console
,{
). - Syntax Analysis: The tokens are analyzed for syntactic correctness and organized into an AST, which represents the code’s structure.
- Lexical Analysis: The code is broken into tokens (e.g.,
- The engine reads the source code and converts it into an Abstract Syntax Tree (AST) through two stages:
- Bytecode Generation
- The AST is translated into bytecode, an intermediate, low-level representation of the code. Bytecode is more efficient for execution than raw JavaScript.
- Execution
- The engine executes the bytecode, initially through an interpreter, which processes instructions step by step.
- Optimization with JIT Compilation
- Modern engines use Just-In-Time (JIT) compilation to improve performance:
- The interpreter identifies frequently executed code paths (“hot code”).
- The JIT compiler converts these paths into machine code, which runs directly on the CPU, making execution significantly faster.
- Engines like V8 have multiple optimization tiers to balance performance and resource use.
- Modern engines use Just-In-Time (JIT) compilation to improve performance:
3. Execution Contexts and Call Stack
The JavaScript engine organizes code execution using:
- Execution Contexts:
- A context is created whenever JavaScript runs, containing:
- Variable Environment: Tracks declared variables and their values.
- Lexical Environment: Tracks scope relationships.
this
Binding: Determines the value ofthis
in the current context.
- The global execution context is created when the script starts, and new contexts are added for functions as they’re called.
- A context is created whenever JavaScript runs, containing:
- Call Stack:
- The call stack is a data structure that tracks function calls in the order they’re invoked.
- Functions are pushed onto the stack when called and popped off when they return.
4. Asynchronous Execution and Event Loop
JavaScript is single-threaded but can handle asynchronous tasks efficiently:
- Web APIs:
- Tasks like
setTimeout
,fetch
, and event listeners are handled by the browser’s APIs or runtime-specific APIs (in Node.js).
- Tasks like
- Callback Queue and Event Loop:
- When an asynchronous operation completes, its callback is queued in the callback queue.
- The event loop continuously checks if the call stack is empty. If so, it pushes queued callbacks onto the stack for execution.
5. Memory Management and Garbage Collection
The engine manages memory using automated garbage collection:
- It identifies unused objects and deallocates memory to prevent leaks.
- Most engines use the mark-and-sweep algorithm, which marks reachable objects and removes unreferenced ones.
Example Execution Flow
Code:
console.log("Start");
setTimeout(() => {
console.log("Async Task");
}, 1000);
console.log("End");
Execution:
- Parsing: The code is parsed into an AST and converted to bytecode.
- Execution:
console.log("Start")
is executed, outputtingStart
.setTimeout
schedules the callback for 1 second and continues without waiting.console.log("End")
is executed, outputtingEnd
.
- Event Loop:
- After 1 second, the
setTimeout
callback (console.log("Async Task")
) is pushed to the call stack and executed, outputtingAsync Task
.
- After 1 second, the
Output:
Start
End
Async Task
6. Optimization techniques
JavaScript engines use advanced optimizations for speed:
- Inline Caching: Caches how object properties are accessed for faster reuse.
- Hidden Classes: Dynamically assigns internal classes to objects for efficient property access.
- Lazy Parsing: Parses unused code only when needed.
- Deoptimization: Reverts optimized machine code to slower bytecode if runtime behavior changes unexpectedly.
This is how JavaScript works under the hood. This comprehensive process highlights JavaScript’s flexibility and the sophisticated mechanisms that power its execution.
Key Features of JavaScript
JavaScript is an essential tool in the modern web developer’s toolkit. As the language that powers interactive web pages and applications, it has many features that make it both powerful and flexible. This guide covers key features of JavaScript, making it accessible for beginners while providing in-depth information for experienced developers.
1. Client-Side Execution
JavaScript is primarily known for its client-side execution. This means JavaScript runs directly in the browser, enabling instant feedback and interactivity without needing server-side processing. This reduces the load on the server, as many tasks, such as form validation, DOM manipulation, and UI updates, can be handled on the client side.
Example:
document.querySelector("button").addEventListener("click", () => {
alert("Button clicked!");
});
2. Platform Independence
JavaScript is platform-independent, meaning it works across all devices and operating systems as long as there is a compatible browser. Whether you’re on Windows, macOS, Linux, or a smartphone, JavaScript provides a consistent experience, making it a universally accessible language.
3. Versatility: Frontend & Backend
JavaScript’s versatility extends beyond the browser. With technologies like Node.js, JavaScript can also run on the server side. This enables developers to use a single language for both client-side and server-side development, which simplifies the development process and promotes code reuse.
- Frontend: Manipulating the DOM to create dynamic, responsive web pages.
- Backend: Building APIs, handling server logic, and creating real-time applications with Node.js.
4. Event-Driven Programming
JavaScript is an event-driven language. This means that actions, such as clicks, form submissions, or keypresses, can trigger specific functions in your code. This allows developers to build highly interactive applications, such as those used in web forms, games, and dynamic interfaces.
document.querySelector("button").addEventListener("click", () => {
alert("Button clicked!");
});
5. Dynamic Typing
JavaScript is dynamically typed, meaning variables do not have a fixed type. The type of a variable is determined at runtime based on the value assigned to it. This gives developers more flexibility but requires careful handling to avoid bugs related to unexpected types.
let variable = 42; // Number
console.log(typeof variable); // "number"
variable = "Hello"; // Now a string
console.log(typeof variable); // "string"
6. Asynchronous Programming
JavaScript supports asynchronous programming, allowing tasks to run without blocking the main thread. This is particularly useful for tasks such as fetching data from a server, reading files, or handling user input. JavaScript uses callbacks, promises, and async/await
syntax to handle asynchronous operations.
Example using async/await
:
async function fetchData() {
const response = await fetch("https://api.example.com/data");
const data = await response.json();
console.log(data);
}
fetchData();
7. Rich Standard Library
JavaScript comes with a comprehensive standard library that provides many built-in functions and objects. This makes it easy to manipulate strings, handle dates, perform mathematical operations, and more, without needing third-party libraries.
const date = new Date();
console.log(date.toDateString()); // Outputs current date
const numbers = [1, 2, 3, 4, 5];
const squared = numbers.map(num => num * num);
console.log(squared); // [1, 4, 9, 16, 25]
8. Object-Oriented and Functional Programming
JavaScript supports both object-oriented programming (OOP) and functional programming paradigms. With OOP, you can create classes, use inheritance, and design systems with objects. Additionally, JavaScript allows for higher-order functions, which enables developers to treat functions as first-class objects.
Example of OOP:
class Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log(`${this.name} makes a sound.`);
}
}
class Dog extends Animal {
speak() {
console.log(`${this.name} barks.`);
}
}
const dog = new Dog("Buddy");
dog.speak(); // Buddy barks.
9. DOM Manipulation
One of JavaScript’s core features is its ability to manipulate the DOM (Document Object Model). The DOM is a representation of a web page’s structure, and JavaScript can modify it, update content, and create dynamic elements on the page. This allows web pages to be interactive without requiring a reload.
Example:
document.querySelector("h1").textContent = "Welcome to JavaScript!";
10. Browser Compatibility
JavaScript is supported by all major browsers, and tools like Babel ensure compatibility with older browser versions. This ensures that your JavaScript-powered applications can reach the widest audience possible, even on outdated browsers.
11. Extensive APIs
JavaScript provides access to a wide variety of built-in APIs, which extend its functionality far beyond simple scripting. Some popular APIs include:
- Web APIs: These allow JavaScript to interact with browser features, such as DOM manipulation, Fetch API, and Local Storage.
- Geolocation API: Access the user’s geographical location.
- Canvas API: Draw graphics and animations.
- WebRTC API: Enable real-time communication between browsers.
Example of using the Geolocation API:
navigator.geolocation.getCurrentPosition((position) => {
console.log(position.coords.latitude, position.coords.longitude);
});
12. Community and Ecosystem
JavaScript has one of the largest and most active developer communities. There are countless open-source libraries, frameworks, and tools available, which simplify development and enhance functionality. Some of the most popular frameworks and libraries include:
- React: A JavaScript library for building user interfaces.
- Angular: A robust framework for building dynamic, single-page applications.
- Vue.js: A lightweight framework for building interactive user interfaces.
Additionally, the Node.js ecosystem is vast, providing packages for everything from web frameworks (like Express.js) to real-time communication (Socket.io).
13. Modules
JavaScript allows you to modularize your code by splitting it into separate files. This helps keep your codebase organized, maintainable, and scalable. Using the import
and export
keywords, you can load and share functionality between different parts of your application.
Example:
// file1.js
export const greet = (name) => `Hello, ${name}`;
// file2.js
import { greet } from './file1.js';
console.log(greet('Alice')); // Output: Hello, Alice!
14. Error Handling (Try/Catch)
JavaScript offers try/catch
blocks for graceful error handling. This enables developers to anticipate and manage potential runtime errors, improving the robustness and reliability of applications.
Example:
try {
let result = riskyFunction();
} catch (error) {
console.log('Error occurred: ', error);
}
JavaScript syntax and basic concepts
JavaScript is one of the most widely used programming languages for building dynamic web applications. Its flexibility, simplicity, and extensive ecosystem make it an essential tool for developers. To get started with JavaScript, it’s important to understand its syntax and basic concepts. This guide will walk you through the fundamental building blocks of JavaScript, ensuring you have a strong foundation for further learning.
1. Variables and constants
Variables in JavaScript are used to store data that can be accessed and modified throughout the program. You can define variables using var
, let
, or const
:
var
: The traditional way to declare variables (older syntax, less commonly used now).let
: Used to declare variables that can be reassigned.const
: Used for constants, meaning the value cannot be reassigned after declaration.
let name = "John";
const age = 25;
var isActive = true;
2. Data types
JavaScript supports several data types that fall into two categories: primitive and non-primitive.
Primitive data types:
- String: Text, e.g.,
"Hello"
- Number: Integers or decimals, e.g.,
42
,3.14
- Boolean: True or false values, e.g.,
true
- Undefined: A variable that has been declared but not assigned a value.
- Null: Represents the intentional absence of any object value.
- Symbol: Unique and immutable identifiers.
Non-primitive data types:
- Object: Used to store collections of data.
- Array: A list of values, e.g.,
[1, 2, 3]
.
let greeting = "Hello, world!"; // String
let count = 10; // Number
let isAdmin = false; // Boolean
let user; // Undefined
let address = null; // Null
let colors = ["red", "blue", "green"]; // Array
let userInfo = { name: "John", age: 25 }; // Object
3. Operators
JavaScript includes a variety of operators for performing calculations, comparisons, and logical operations.
- Arithmetic Operators:
+
,-
,*
,/
,%
(modulus) - Assignment Operators:
=
,+=
,-=
,*=
,/=
- Comparison Operators:
==
,===
,!=
,!==
,<
,>
,<=
,>=
- Logical Operators:
&&
(AND),||
(OR),!
(NOT)
let x = 10;
x += 5; // x is now 15
let isGreater = x > 10; // true
let isValid = isGreater && x < 20; // true
4. Conditional statements
Conditional statements allow you to execute code based on certain conditions. Common structures include if
, else
, else if
, and switch
statements.
let score = 85;
if (score >= 90) {
console.log("Grade: A");
} else if (score >= 75) {
console.log("Grade: B");
} else {
console.log("Grade: C");
}
5. Loops
Loops allow you to execute a block of code multiple times. JavaScript offers several types of loops:
for
loop: Repeats a block of code a specific number of times.while
loop: Executes as long as a condition is true.do...while
loop: Executes the block of code at least once, then continues while the condition is true.for...of
loop: Iterates over iterable objects like arrays.for...in
loop: Iterates over the properties of an object.
for (let i = 0; i < 5; i++) {
console.log(i); // Outputs 0, 1, 2, 3, 4
}
let colors = ["red", "green", "blue"];
for (let color of colors) {
console.log(color); // Outputs each color in the array
}
6. Functions
Functions are reusable blocks of code designed to perform specific tasks. They can accept parameters and return values.
function greet(name) {
return `Hello, ${name}!`;
}
console.log(greet("Alice")); // Outputs: Hello, Alice!
JavaScript also supports arrow functions, which provide a more concise syntax:
const multiply = (a, b) => a * b;
console.log(multiply(3, 4)); // Outputs: 12
7. Events and event listeners
JavaScript enables interactivity on web pages through events like clicks, key presses, or mouse movements. Event listeners detect these actions and execute specific code in response.
const button = document.querySelector("button");
button.addEventListener("click", () => {
alert("Button clicked!");
});
8. The document object model (DOM)
The DOM represents the structure of a web page. JavaScript can manipulate the DOM to update content dynamically.
const heading = document.querySelector("h1");
heading.textContent = "Welcome to JavaScript!";
heading.style.color = "blue";
Versatile roles of JavaScript across the development spectrum
1. Client-side scripting
JavaScript plays a vital role in client-side scripting, enabling dynamic and interactive web pages. Developers can manipulate HTML and CSS to create content that updates in real-time without reloading the page. This includes validating form inputs to ensure users submit correct data and creating engaging animations. Event handling—responding to user actions such as clicks, keypresses, or mouse movements—is another essential feature. Additionally, JavaScript’s ability to manipulate the Document Object Model (DOM) allows developers to dynamically update, delete, or rearrange elements on a webpage, significantly improving user experience.
2. Server-side programming
On the server side, JavaScript has become a powerful tool thanks to Node.js. It is used to build backend applications that handle user requests, process data, and interact with databases. Developers can create APIs and web services using frameworks like Express.js, enabling seamless communication between the front end and the server. Real-time applications such as chat apps and live notifications are powered by JavaScript frameworks like Socket.IO, allowing for instantaneous data exchange and enhanced user engagement. Its versatility makes JavaScript a go-to language for full-stack development.
3. Full-stack development
JavaScript simplifies full-stack development by offering solutions for both client-side and server-side programming. Frontend frameworks such as React, Angular, and Vue.js allow developers to build intuitive and responsive user interfaces. On the backend, Node.js and Express.js manage server logic and database interactions. By using JavaScript across the entire stack, developers streamline their workflows and reduce the need for multiple languages, resulting in faster development cycles and cohesive application architecture.
4. Building progressive web applications (PWAs)
Progressive Web Applications (PWAs) use JavaScript to deliver app-like experiences within a browser. Service Workers, a core JavaScript feature, enable offline functionality by caching key resources, ensuring users can access content even without an internet connection. JavaScript also facilitates push notifications, helping businesses engage users with timely updates. PWAs combine the best of web and mobile apps, offering fast load times, smooth navigation, and seamless cross-device compatibility.
5. Game development
JavaScript has made game development accessible to web developers, offering tools and libraries to create both 2D and 3D games. Frameworks like Phaser provide the foundation for developing engaging 2D games, while libraries like Three.js and Babylon.js enable the creation of immersive 3D environments. JavaScript also supports physics engines and basic artificial intelligence, helping developers build realistic game mechanics and interactive experiences that run smoothly in web browsers.
6. Mobile app development
With frameworks like React Native, Ionic, and NativeScript, JavaScript extends its capabilities to mobile app development. These frameworks allow developers to build cross-platform apps using a single codebase, reducing development time and effort. JavaScript-based mobile apps can access native device features such as cameras, GPS, and push notifications, providing users with seamless and engaging experiences comparable to native apps built with platform-specific languages.
7. Desktop application development
JavaScript is also used to build desktop applications that work across multiple platforms. Frameworks like Electron enable developers to create desktop apps using web technologies like HTML, CSS, and JavaScript. This approach allows for faster development and maintenance while ensuring compatibility with Windows, macOS, and Linux. Popular applications like Slack, Visual Studio Code, and Discord demonstrate the power and versatility of JavaScript in the desktop application space.
8. Automation and scripting
JavaScript is a popular choice for automating repetitive tasks and scripting workflows. Tools like Puppeteer and Playwright enable developers to automate browser-based tasks such as testing, web scraping, and form submissions. JavaScript can also be used for workflow automation, simplifying tasks like data transformation, file generation, and deployment processes. This flexibility makes it an invaluable tool for improving productivity and reducing manual effort.
9. Data visualization
Data visualization is another area where JavaScript excels, offering libraries like D3.js, Chart.js, and Highcharts to create interactive and visually appealing charts, graphs, and dashboards. These tools enable developers to turn complex datasets into actionable insights by presenting information in an easily understandable format. JavaScript-powered data visualizations are widely used in analytics platforms, financial reports, and performance dashboards to enhance decision-making processes.
10. AI and Machine learning
JavaScript is becoming increasingly popular in the fields of artificial intelligence and machine learning. Libraries like TensorFlow.js and Brain.js allow developers to train and deploy machine learning models directly in the browser. Applications include image recognition, sentiment analysis, and natural language processing. By leveraging JavaScript, developers can integrate AI features into web applications without requiring additional software, making advanced technology accessible to a broader audience.
11. Internet of things (IoT)
JavaScript is also used in Internet of Things (IoT) development, enabling devices to communicate with each other and with the cloud. Frameworks like Johnny-Five allow developers to control hardware components such as sensors, actuators, and LEDs using JavaScript. This makes it possible to build smart devices, automate home systems, and monitor industrial equipment. JavaScript’s simplicity and extensive ecosystem make it an excellent choice for IoT projects.
12. Browser extensions
JavaScript powers browser extensions that enhance user experiences by adding new features and functionality to web browsers. Extensions like ad blockers, password managers, and content enhancers rely on JavaScript to modify webpage content, handle user interactions, and store data securely. By leveraging JavaScript’s ability to interact with the DOM and browser APIs, developers can create powerful tools that improve productivity and browsing efficiency.
13. Web assembly (WASM) Integration
WebAssembly (WASM) allows JavaScript to work alongside high-performance languages like C++ and Rust, enabling resource-intensive applications to run efficiently in the browser. With WASM, developers can create advanced applications such as video editors, games, and scientific simulations that demand high computational power. JavaScript acts as a bridge, integrating these applications into web environments and making them accessible without requiring users to install additional software.
Step-by-step guide for getting started with JavaScript on your PC
JavaScript is a powerful programming language used for web development and much more. If you’re looking to start learning JavaScript on your PC, this step-by-step guide will help you set up your environment and write your first program.
Step 1: Install a Text Editor
To write JavaScript code, you’ll need a text editor. Here are some popular options:
- Visual Studio Code (VS Code): A free, feature-rich editor. Download it from https://code.visualstudio.com/.
- Notepad++: Lightweight and simple. Download it from https://notepad-plus-plus.org/.
- Sublime Text: A sleek editor with great features. Download it from https://www.sublimetext.com/.
Recommended: Install Visual Studio Code
- Go to the Visual Studio Code website.
- Download the installer for your operating system.
- Run the installer and follow the setup instructions.
Step 2: Install a Web Browser
JavaScript runs in web browsers, so you’ll need one installed. Most modern browsers come with built-in developer tools for JavaScript. Popular browsers include:
- Google Chrome
- Mozilla Firefox
- Microsoft Edge
- Safari (for macOS)
Recommended: Use Google Chrome
- Go to https://www.google.com/chrome/.
- Download and install the browser.
Step 3: Open the Developer Tools
Modern browsers have developer tools that allow you to test JavaScript directly. Here’s how to access them:
- Open your browser.
- Press
Ctrl + Shift + J
(Windows) orCmd + Option + J
(Mac) to open the console in Chrome or Edge. - For Firefox, press
Ctrl + Shift + K
(Windows) orCmd + Option + K
(Mac).
The console is where you can write and execute JavaScript code immediately.
Step 4: Create Your First JavaScript Program
Let’s write a simple JavaScript program that prints a message to the console. Follow these steps:
Create an HTML File
- Open your text editor.
- Create a new file and save it as
index.html
. - Add the following code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript Demo</title>
</head>
<body>
<h1>Hello, JavaScript!</h1>
<script src="script.js"></script>
</body>
</html>
Create a JavaScript File
- In the same folder as
index.html
, create a new file namedscript.js
. - Add the following code to
script.js
:
console.log('Hello, JavaScript!');
Step 5: Run Your Program
- Open the folder where you saved
index.html
andscript.js
. - Right-click on
index.html
and select “Open with” > your web browser. - Open the developer tools console (see Step 3).
- You’ll see the message
Hello, JavaScript!
printed in the console.
Step 6: Practice and Experiment
Now that your environment is set up, you can start experimenting with JavaScript. Try modifying the code in script.js
to:
- Perform calculations:
console.log(5 + 3);
- Create a function:
function greet(name) {
console.log(`Hello, ${name}!`);
}
greet('Alice');
- Manipulate the HTML content:
document.querySelector('h1').textContent = 'Welcome to JavaScript!';
Conclusion
Congratulations! You’ve set up your PC to write and run JavaScript code. By following this guide, you’ve taken your first steps toward mastering one of the most in-demand programming languages.
JavaScript is an essential tool for web developers. Its versatility, ease of use, and widespread adoption make it a powerful language for building engaging and functional web experiences. Whether you’re a beginner or an experienced developer, mastering JavaScript can open up countless opportunities in the tech world.
JavaScript works seamlessly with browsers to deliver dynamic and interactive web experiences. Its execution involves parsing, JIT compilation, and efficient memory management, all handled by robust JavaScript engines. Understanding how JavaScript works is key to mastering web development and creating high-performing applications.
Keep practicing and building small projects to enhance your skills.
Get up to 85% off
Build your project using Managed VPS & Dedicated hosting and decrease your workload.
You'll get seamless migration, exceptional savings, 24/7 technical support, HIPAA compliant, PCI compliant, Multi-Level DDoS protection, 30-day money-back guarantee and more with Liquid Web.
Affiliate Disclosure: Affiliate links are available here. If you click through the affiliate links and buy something, we may get a small commission, and you'll not be charged extra.