JavaScript Comments: A Comprehensive Guide for beginners
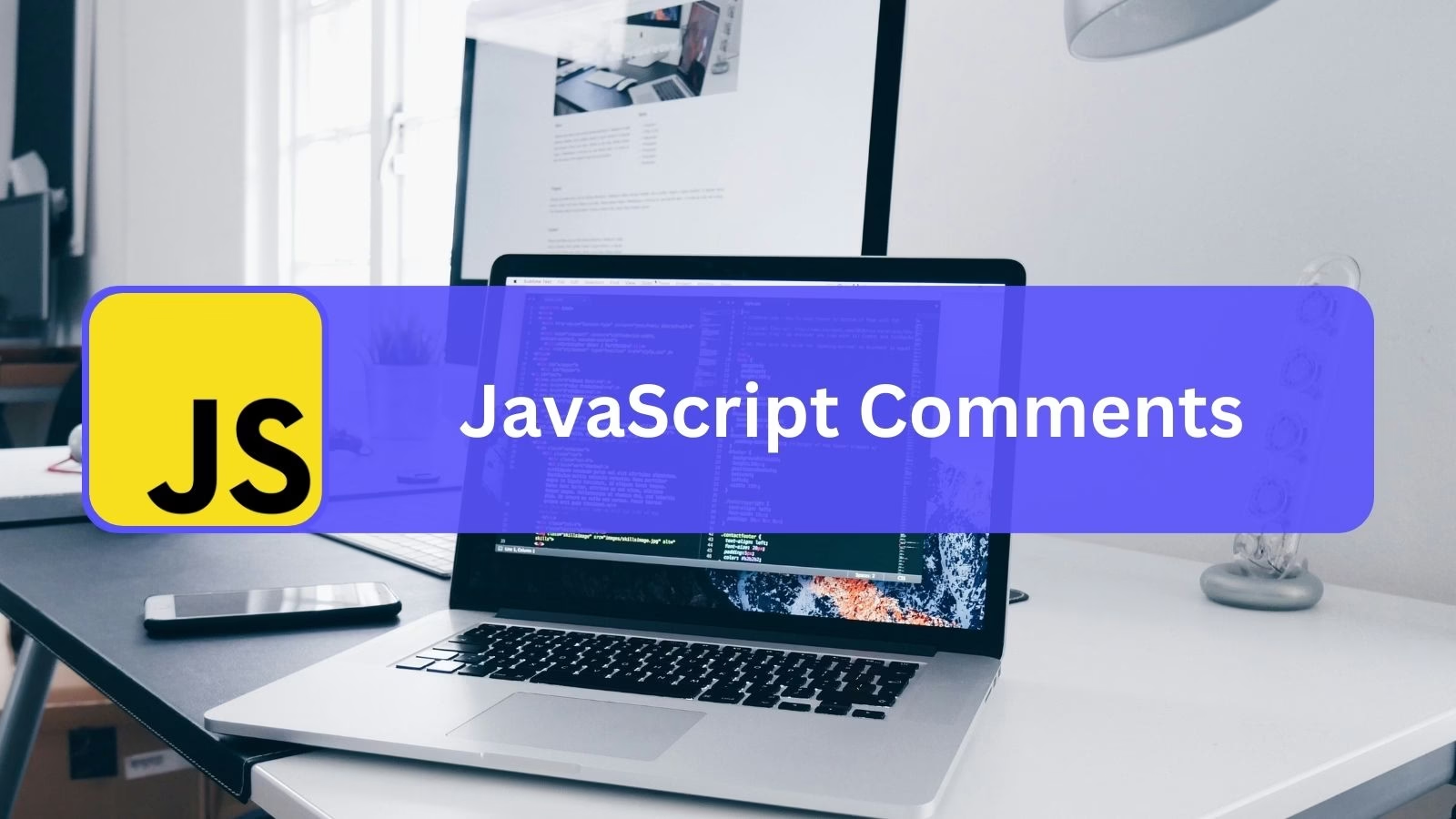
This post was generated with AI and then edited by us.
Comments in JavaScript are an essential tool for developers. They help document code, clarify functionality, and improve collaboration within a team. In this blog post, we will explore the different types of comments in JavaScript, their purposes, and best practices for using them effectively.
What are JavaScript comments?
Comments are lines of code that JavaScript ignores during execution. They are used to provide explanations or annotate sections of code without affecting the program’s functionality. This helps make code more readable and maintainable.
Types of comments in JavaScript
JavaScript supports two types of comments:
1. Single-line Comments
Single-line comments start with //
. Everything following //
on the same line is treated as a comment.
Example:
// This is a single-line comment
let x = 5; // Declare a variable x and assign it the value 5
Single-line comments are often used for brief explanations or inline annotations.
2. Multiline Comments
Multi-line comments start with /*
and end with */
. They can span multiple lines and are used for longer explanations or to temporarily disable blocks of code.
Example:
/*
This is a multi-line comment.
It can be used to explain complex logic,
provide detailed documentation, or temporarily disable code.
*/
let y = 10;
Multi-line comments are ideal for providing detailed descriptions or commenting out larger code sections.
Why use comments in JavaScript?
Comments serve several important purposes:
- Enhance Code Readability: Comments make your code easier to understand for yourself and others.
- Document Functionality: Clearly explain the purpose of functions, variables, and classes.
- Debugging Assistance: Temporarily disable parts of your code without deleting them.
- Collaboration: Facilitate teamwork by providing context and insights into the code.
Best practices for writing comments
To maximize the benefits of comments, follow these best practices:
1. Keep Comments Relevant and Concise Avoid over-commenting. Write comments that add value and clarify the code’s intent.
2. Use Comments to Explain Why, Not What Focus on explaining why certain decisions were made rather than describing what the code does. Code should generally be self-explanatory.
Example:
// Using a set to ensure unique values
const uniqueItems = new Set(array);
3. Avoid Commenting Out Large Blocks of Code If you need to remove code, consider using version control systems like Git instead of leaving commented-out code in your files.
4. Adopt a Consistent Style Use a consistent style for comments across your codebase to maintain readability and professionalism.
5. Use Comments Sparingly Too many comments can clutter your code. Write clean and clear code that requires minimal explanation.
Common use cases for comments
1. Annotating Functions and Classes Describe the purpose and usage of functions and classes.
/**
* Calculates the factorial of a number.
* @param {number} n - The number to calculate the factorial for.
* @returns {number} The factorial of the number.
*/
function factorial(n) {
if (n === 0) return 1;
return n * factorial(n - 1);
}
2. Documenting Complex Logic Provide context for intricate algorithms or processes.
// Sorting the array in descending order
array.sort((a, b) => b - a);
3. Flagging TODOs and Fixes Use comments to indicate pending tasks or issues.
// TODO: Optimize this loop for performance
for (let i = 0; i < array.length; i++) {
process(array[i]);
}
Tools to improve commenting
- Linters: Tools like ESLint can enforce comment guidelines.
- Documentation Generators: Tools like JSDoc help create comprehensive documentation from comments.
Conclusion
Comments are a powerful yet often overlooked aspect of JavaScript development. By using them effectively, you can create code that is not only functional but also maintainable and collaborative. Remember to write comments with clarity and purpose, ensuring they add value to your codebase.
Get up to 85% off
Build your project using Managed VPS & Dedicated hosting and decrease your workload.
You'll get seamless migration, exceptional savings, 24/7 technical support, HIPAA compliant, PCI compliant, Multi-Level DDoS protection, 30-day money-back guarantee and more with Liquid Web.
Affiliate Disclosure: Affiliate links are available here. If you click through the affiliate links and buy something, we may get a small commission, and you'll not be charged extra.