JavaScript Operators: A Comprehensive Guide for Beginners
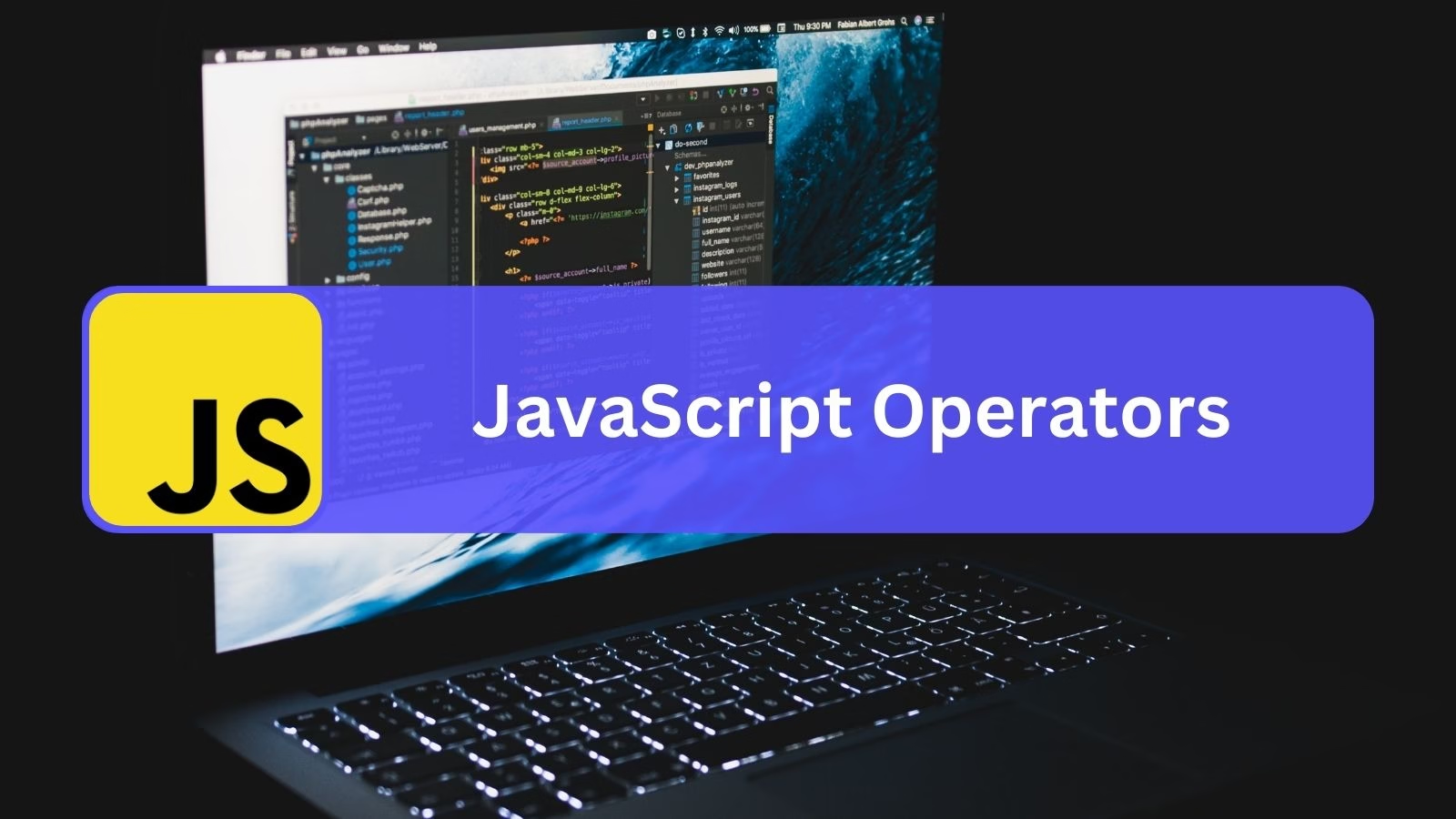
This post was generated with AI and then edited by us.
JavaScript is a powerful and versatile programming language that plays a crucial role in web development. From creating dynamic web pages to handling complex computations, JavaScript is an essential tool for developers.
One of the fundamental aspects of JavaScript is its operators, which enable you to perform various operations on variables and values. Understanding these operators is key to writing efficient and effective JavaScript code.
In this comprehensive guide, we will explore different types of JavaScript operators. Through clear explanations and simple code examples, this guide aims to equip beginners with the knowledge they need to master JavaScript operators and enhance their coding skills.
Types of JavaScript Operators:
- Arithmetic Operators
- Assignment Operators
- Comparison Operators
- Logical Operators
- Bitwise Operators
- Conditional (Ternary) Operator
- String Operators
- Type Operators
Arithmetic Operators in JavaScript
Arithmetic operators are used to perform basic mathematical operations. These operators allow you to add, subtract, multiply, divide, and find the remainder of numbers. They are fundamental for any type of numerical calculations in JavaScript. Here’s a closer look at each arithmetic operator:
1. Addition (+
)
The addition operator adds two values.
let a = 10;
let b = 3;
let result = a + b; // Output: 13
console.log(result); // Output: 13
2. Subtraction (-
)
The subtraction operator subtracts one value from another.
let a = 10;
let b = 3;
let result = a - b; // Output: 7
console.log(result); // Output: 7
3. Multiplication (*
)
The multiplication operator multiplies two values.
let a = 10;
let b = 3;
let result = a * b; // Output: 30
console.log(result); // Output: 30
4. Division (/
)
The division operator divides one value by another.
let a = 10;
let b = 3;
let result = a / b; // Output: 3.3333
console.log(result); // Output: 3.3333
5. Modulus (%
)
The modulus operator returns the remainder of a division.
let a = 10;
let b = 3;
let result = a % b; // Output: 1
console.log(result); // Output: 1
6. Exponentiation (**
)
The exponentiation operator raises the first value to the power of the second value.
let a = 10;
let b = 3;
let result = a ** b; // Output: 1000
console.log(result); // Output: 1000
Summary Table
Operator | Description | Example | Output |
---|---|---|---|
+ | Addition | 10 + 3 | 13 |
- | Subtraction | 10 - 3 | 7 |
* | Multiplication | 10 * 3 | 30 |
/ | Division | 10 / 3 | 3.3333 |
% | Modulus | 10 % 3 | 1 |
** | Exponentiation | 10 ** 3 | 1000 |
Practical Example
Let’s combine these arithmetic operators in a practical example:
let x = 5;
let y = 2;
let z = 10;
let sum = x + y; // 5 + 2 = 7
let difference = z - y; // 10 - 2 = 8
let product = x * y; // 5 * 2 = 10
let quotient = z / y; // 10 / 2 = 5
let remainder = z % y; // 10 % 2 = 0
let power = x ** y; // 5 ** 2 = 25
console.log(sum); // Output: 7
console.log(difference); // Output: 8
console.log(product); // Output: 10
console.log(quotient); // Output: 5
console.log(remainder); // Output: 0
console.log(power); // Output: 25
Assignment Operators in JavaScript
Assignment operators are used to assign values to variables. They not only assign the initial value to a variable but can also perform operations and then assign the result back to the variable. These operators are essential for manipulating and updating variable values efficiently.
1. Assignment (=
)
The assignment operator assigns the value on the right to the variable on the left.
let x = 5;
console.log(x); // Output: 5
2. Addition Assignment (+=
)
The addition assignment operator adds the value on the right to the variable and assigns the result back to the variable.
let x = 5;
x += 3; // Equivalent to x = x + 3
console.log(x); // Output: 8
3. Subtraction Assignment (-=
)
The subtraction assignment operator subtracts the value on the right from the variable and assigns the result back to the variable.
let x = 5;
x -= 2; // Equivalent to x = x - 2
console.log(x); // Output: 3
4. Multiplication Assignment (*=
)
The multiplication assignment operator multiplies the variable by the value on the right and assigns the result back to the variable.
let x = 5;
x *= 4; // Equivalent to x = x * 4
console.log(x); // Output: 20
5. Division Assignment (/=
)
The division assignment operator divides the variable by the value on the right and assigns the result back to the variable.
let x = 5;
x /= 2; // Equivalent to x = x / 2
console.log(x); // Output: 2.5
6. Modulus Assignment (%=
)
The modulus assignment operator takes the remainder of dividing the variable by the value on the right and assigns it back to the variable.
let x = 5;
x %= 2; // Equivalent to x = x % 2
console.log(x); // Output: 1
Summary Table
Operator | Description | Example | Equivalent to | Result |
---|---|---|---|---|
= | Assignment | x = 5 | x = 5 | 5 |
+= | Addition Assignment | x += 3 | x = x + 3 | 8 |
-= | Subtraction Assignment | x -= 2 | x = x - 2 | 3 |
*= | Multiplication Assignment | x *= 4 | x = x * 4 | 20 |
/= | Division Assignment | x /= 2 | x = x / 2 | 2.5 |
%= | Modulus Assignment | x %= 2 | x = x % 2 | 1 |
Practical Example
Let’s use these assignment operators in a practical example:
let total = 100;
let discount = 20;
total -= discount; // Subtract discount from total
console.log(total); // Output: 80
total *= 2; // Double the total
console.log(total); // Output: 160
total /= 4; // Divide the total by 4
console.log(total); // Output: 40
total %= 6; // Get the remainder when total is divided by 6
console.log(total); // Output: 4
Explanation:
total -= discount
subtracts the value ofdiscount
(20) fromtotal
(100), resulting in 80.total *= 2
multipliestotal
by 2, resulting in 160.total /= 4
dividestotal
by 4, resulting in 40.total %= 6
takes the remainder oftotal
divided by 6, resulting in 4.
Comparison Operators in JavaScript
Comparison operators are used to compare two values and return a boolean result (true
or false
). They are essential for making decisions in your code, allowing you to execute different code blocks based on certain conditions. Here are the main comparison operators in JavaScript:
1. Equal to (==
)
The ==
operator checks if two values are equal in value, regardless of their type.
let a = 7;
let b = '7';
console.log(a == b); // Output: true
2. Strict Equal to (===
)
The ===
operator checks if two values are equal in value and type.
let a = 7;
let b = '7';
console.log(a === b); // Output: false
3. Not Equal to (!=
)
The !=
operator checks if two values are not equal in value.
let a = 7;
let b = '7';
console.log(a != b); // Output: false
4. Strict Not Equal to (!==
)
The !==
operator checks if two values are not equal in value and type.
let a = 7;
let b = '7';
console.log(a !== b); // Output: true
5. Greater than (>
)
The >
operator checks if the value on the left is greater than the value on the right.
let a = 10;
let b = 5;
console.log(a > b); // Output: true
6. Greater than or Equal to (>=
)
The >=
operator checks if the value on the left is greater than or equal to the value on the right.
let a = 10;
let b = 10;
console.log(a >= b); // Output: true
7. Less than (<
)
The <
operator checks if the value on the left is less than the value on the right.
let a = 5;
let b = 10;
console.log(a < b); // Output: true
8. Less than or Equal to (<=
)
The <=
operator checks if the value on the left is less than or equal to the value on the right.
let a = 5;
let b = 5;
console.log(a <= b); // Output: true
Summary Table
Operator | Description | Example | Output |
---|---|---|---|
== | Equal to | 7 == '7' | true |
=== | Strict Equal to | 7 === '7' | false |
!= | Not Equal to | 7 != '7' | false |
!== | Strict Not Equal to | 7 !== '7' | true |
> | Greater than | 10 > 5 | true |
>= | Greater than or Equal to | 10 >= 10 | true |
< | Less than | 5 < 10 | true |
<= | Less than or Equal to | 5 <= 5 | true |
Practical Example
Let’s see a practical example that uses multiple comparison operators:
let age = 20;
let isAdult = age >= 18; // Checks if age is 18 or older
console.log(isAdult); // Output: true
let temperature = 30;
let isCold = temperature < 15; // Checks if temperature is less than 15
console.log(isCold); // Output: false
let password = 'securePassword';
let confirmPassword = 'securePassword';
let isMatch = password === confirmPassword; // Checks if both passwords match
console.log(isMatch); // Output: true
Explanation:
isAdult
checks ifage
is greater than or equal to 18. Sinceage
is 20, the result istrue
.isCold
checks iftemperature
is less than 15. Sincetemperature
is 30, the result isfalse
.isMatch
checks ifpassword
andconfirmPassword
are strictly equal. Since both are'securePassword'
, the result istrue
.
Logical Operators in JavaScript
Logical operators are used to combine or invert boolean values (true
or false
). They are fundamental in making decisions and controlling the flow of a program by evaluating multiple conditions. Let’s explore the main logical operators in JavaScript:
1. Logical AND (&&
)
The logical AND operator returns true
if both operands are true
. If one or both operands are false
, it returns false
.
let a = true;
let b = false;
console.log(a && b); // Output: false
console.log(a && true); // Output: true
console.log(false && b); // Output: false
2. Logical OR (||
)
The logical OR operator returns true
if at least one of the operands is true
. If both operands are false
, it returns false
.
let a = true;
let b = false;
console.log(a || b); // Output: true
console.log(a || true); // Output: true
console.log(false || b); // Output: false
3. Logical NOT (!
)
The logical NOT operator inverts the boolean value. It returns true
if the operand is false
, and false
if the operand is true
.
let a = true;
let b = false;
console.log(!a); // Output: false
console.log(!b); // Output: true
Summary Table
Operator | Description | Example Expression | Result | Explanation |
---|---|---|---|---|
&& | Logical AND | false && false | false | Both operands are false , so it returns false . |
&& | Logical AND | true && false | false | Both operands must be true to return true . |
&& | Logical AND |
| true | Both operands are true , so it returns true . |
|| | Logical OR |
| true | At least one operand is true , so it returns true . |
|| | Logical OR | true || false | true | At least one operand is true , so it returns true . |
|| | Logical OR | false || false | false | Both operands are false , so it returns false . |
! | Logical NOT | !true | false | Inverts true to false . |
! | Logical NOT | !false | true | Inverts false to true . |
Practical Example
Let’s look at a practical example that uses all three logical operators:
let age = 20;
let hasPermission = true;
// Logical AND
if (age >= 18 && hasPermission) {
console.log("Access granted."); // Output: Access granted.
} else {
console.log("Access denied.");
}
// Logical OR
if (age >= 18 || hasPermission) {
console.log("Partial access granted."); // Output: Partial access granted.
} else {
console.log("Access denied.");
}
// Logical NOT
if (!hasPermission) {
console.log("Access denied.");
} else {
console.log("Permission granted."); // Output: Permission granted.
}
Explanation:
- Logical AND (
&&
): Checks if bothage >= 18
andhasPermission
are true. Since both are true, it grants access. - Logical OR (
||
): Checks if at least one ofage >= 18
orhasPermission
is true. Since at least one is true, it grants partial access. - Logical NOT (
!
): Inverts the value ofhasPermission
. SincehasPermission
is true,!hasPermission
is false, and it grants permission.
Bitwise Operators in JavaScript
Bitwise operators perform bit-level operations on the binary representations of numbers. These operators are powerful tools for performing low-level calculations and manipulations. Here are the main bitwise operators in JavaScript:
1. Bitwise AND (&
)
The bitwise AND operator returns a 1
in each bit position where both corresponding bits of both operands are 1
.
let a = 5; // Binary: 0101
let b = 3; // Binary: 0011
let result = a & b; // Binary: 0001
console.log(result); // Output: 1
2. Bitwise OR (|
)
The bitwise OR operator returns a 1
in each bit position where at least one of the corresponding bits of either operand is 1
.
let a = 5; // Binary: 0101
let b = 3; // Binary: 0011
let result = a | b; // Binary: 0111
console.log(result); // Output: 7
3. Bitwise XOR (^
)
The bitwise XOR operator returns a 1
in each bit position where only one of the corresponding bits of either operand is 1
.
let a = 5; // Binary: 0101
let b = 3; // Binary: 0011
let result = a ^ b; // Binary: 0110
console.log(result); // Output: 6
4. Bitwise NOT (~
)
The bitwise NOT operator inverts each bit of its operand, turning 0
s into 1
s and 1
s into 0
s (this is also called a bitwise complement).
let a = 5; // Binary: 0101
let result = ~a; // Binary: 1010 (Two's complement for -6)
console.log(result); // Output: -6
5. Bitwise Left Shift (<<
)
The left shift operator shifts the bits of the operand to the left by the specified number of positions, filling the vacant rightmost bits with 0
s.
let a = 5; // Binary: 0101
let result = a << 1; // Binary: 1010
console.log(result); // Output: 10
6. Bitwise Right Shift (>>
)
The right shift operator shifts the bits of the operand to the right by the specified number of positions, discarding the shifted bits.
let a = 5; // Binary: 0101
let result = a >> 1; // Binary: 0010
console.log(result); // Output: 2
7. Bitwise Unsigned Right Shift (>>>
)
The unsigned right shift operator shifts the bits of the operand to the right by the specified number of positions, filling the leftmost bits with 0
s.
let a = 5; // Binary: 0101
let result = a >>> 1; // Binary: 0010
console.log(result); // Output: 2
Summary Table
Operator | Example | Output | Binary Representation |
---|---|---|---|
& | 5 & 3 | 1 | 0101 & 0011 = 0001 |
| | 5 | 3 | 7 | 0101 | 0011 = 0111 |
^ | 5 ^ 3 | 6 | 0101 ^ 0011 = 0110 |
~ | ~5 | -6 | ~0101 = 1010 |
<< | 5 << 1 | 10 | 0101 << 1 = 1010 |
>> | 5 >> 1 | 2 | 0101 >> 1 = 0010 |
>>> | 5 >>> 1 | 2 | 0101 >>> 1 = 0010 |
Practical Example
Let’s combine these bitwise operators in a practical example:
let x = 12; // Binary: 1100
let y = 5; // Binary: 0101
let andResult = x & y; // 1100 & 0101 = 0100 (Output: 4)
let orResult = x | y; // 1100 | 0101 = 1101 (Output: 13)
let xorResult = x ^ y; // 1100 ^ 0101 = 1001 (Output: 9)
let notResult = ~x; // ~1100 = 0011 (Two's complement: -13)
let leftShiftResult = x << 1; // 1100 << 1 = 11000 (Output: 24)
let rightShiftResult = x >> 1; // 1100 >> 1 = 0110 (Output: 6)
let unsignedRightShiftResult = x >>> 1; // 1100 >>> 1 = 0110 (Output: 6)
console.log(andResult); // Output: 4
console.log(orResult); // Output: 13
console.log(xorResult); // Output: 9
console.log(notResult); // Output: -13
console.log(leftShiftResult); // Output: 24
console.log(rightShiftResult); // Output: 6
console.log(unsignedRightShiftResult); // Output: 6
Conditional (Ternary) Operator in JavaScript
The conditional (ternary) operator is a concise way to perform a conditional (if-else) statement in a single line of code. It is the only JavaScript operator that takes three operands, hence the name “ternary.”
Syntax
The syntax for the ternary operator is as follows:
condition ? exprIfTrue : exprIfFalse
condition
: The condition to evaluate.exprIfTrue
: The expression to execute if the condition is true.exprIfFalse
: The expression to execute if the condition is false.
Example
Here is a simple example of how the ternary operator works:
let age = 18;
let canVote = (age >= 18) ? 'Yes' : 'No';
console.log(canVote); // Output: Yes
Explanation:
- The condition
(age >= 18)
checks if the variableage
is greater than or equal to 18. - If the condition is true, the expression
exprIfTrue
(‘Yes’) is executed. - If the condition is false, the expression
exprIfFalse
(‘No’) is executed.
Practical Example
Let’s see a more practical example using the ternary operator:
let score = 85;
let grade = (score >= 90) ? 'A' :
(score >= 80) ? 'B' :
(score >= 70) ? 'C' :
(score >= 60) ? 'D' : 'F';
console.log(`Score: ${score}, Grade: ${grade}`); // Output: Score: 85, Grade: B
Explanation:
- The ternary operator is used to assign a grade based on the score.
- Multiple conditions are chained together to check the score and assign the corresponding grade.
Summary Table
Syntax | Description | Example | Output |
---|---|---|---|
condition ? exprIfTrue : exprIfFalse | Evaluate condition and return either exprIfTrue or exprIfFalse based on the result | (age >= 18) ? 'Yes' : 'No' | Yes or No |
Chained Conditions | Chain multiple conditions to evaluate and return corresponding expressions | (score >= 90) ? 'A' : (score >= 80) ? 'B' : 'F' | A , B , or F |
String Operators in JavaScript
String operators are used to perform operations on strings. They allow you to concatenate (join) strings, compare strings, and perform various manipulations. Here are the main string operators in JavaScript:
1. Concatenation (+
)
The concatenation operator joins two or more strings together.
let firstName = 'John';
let lastName = 'Doe';
let fullName = firstName + ' ' + lastName;
console.log(fullName); // Output: John Doe
2. Concatenation Assignment (+=
)
The concatenation assignment operator appends a string to an existing string variable.
let greeting = 'Hello';
greeting += ', world!';
console.log(greeting); // Output: Hello, world!
Summary Table
Operator | Description | Example | Output |
---|---|---|---|
+ | Concatenation | 'Hello' + ' ' + 'World' | Hello World |
+= | Concatenation Assignment | greeting += ', world!' | Hello, world! |
Practical Example
Let’s combine these string operators in a practical example:
let sentence = 'The quick brown fox';
let action = 'jumps over';
let target = 'the lazy dog';
let fullSentence = sentence + ' ' + action + ' ' + target + '.';
console.log(fullSentence); // Output: The quick brown fox jumps over the lazy dog.
sentence += ' ' + action + ' ' + target + '.';
console.log(sentence); // Output: The quick brown fox jumps over the lazy dog.
Explanation:
fullSentence
uses the+
operator to concatenate multiple strings into one sentence.sentence += ' ' + action + ' ' + target + '.'
appends additional strings to the originalsentence
.
Type Operators in JavaScript
Type operators are used to determine and manipulate the types of variables and values in JavaScript. These operators help you understand what kind of data a variable holds, which is crucial for performing appropriate operations on that data. Here are the key type operators in JavaScript:
1. typeof
Operator
The typeof
operator returns a string indicating the type of the unevaluated operand.
let number = 42;
let string = "Hello, world!";
let boolean = true;
let undefinedValue;
let nullValue = null;
let object = { name: "John" };
let array = [1, 2, 3];
let func = function() { return "Hello!"; };
console.log(typeof number); // Output: "number"
console.log(typeof string); // Output: "string"
console.log(typeof boolean); // Output: "boolean"
console.log(typeof undefinedValue); // Output: "undefined"
console.log(typeof nullValue); // Output: "object" (this is a quirk of JavaScript)
console.log(typeof object); // Output: "object"
console.log(typeof array); // Output: "object" (arrays are considered objects)
console.log(typeof func); // Output: "function"
Explanation:
typeof number
returns"number"
becausenumber
is a numeric value.typeof string
returns"string"
becausestring
is a text value.typeof boolean
returns"boolean"
becauseboolean
is a boolean value (true/false).typeof undefinedValue
returns"undefined"
because the variable is declared but not assigned a value.typeof nullValue
returns"object"
due to a quirk in JavaScript wherenull
is considered an object.typeof object
returns"object"
because it is an object.typeof array
returns"object"
because arrays are also objects in JavaScript.typeof func
returns"function"
becausefunc
is a function.
2. instanceof
Operator
The instanceof
operator tests whether an object is an instance of a particular class or constructor.
class Person {
constructor(name) {
this.name = name;
}
}
let john = new Person("John");
let date = new Date();
console.log(john instanceof Person); // Output: true
console.log(date instanceof Date); // Output: true
console.log(john instanceof Date); // Output: false
console.log(date instanceof Person); // Output: false
Explanation:
john instanceof Person
returnstrue
becausejohn
is an instance of thePerson
class.date instanceof Date
returnstrue
becausedate
is an instance of theDate
class.john instanceof Date
returnsfalse
becausejohn
is not an instance of theDate
class.date instanceof Person
returnsfalse
becausedate
is not an instance of thePerson
class.
3. constructor
Property
The constructor
property returns a reference to the function that created the instance’s prototype.
let array = [1, 2, 3];
let object = { name: "John" };
let func = function() { return "Hello!"; };
console.log(array.constructor === Array); // Output: true
console.log(object.constructor === Object); // Output: true
console.log(func.constructor === Function); // Output: true
Explanation:
array.constructor === Array
returnstrue
because the constructor for the array isArray
.object.constructor === Object
returnstrue
because the constructor for the object isObject
.func.constructor === Function
returnstrue
because the constructor for the function isFunction
.
Summary Table
Operator | Description | Example | Output |
---|---|---|---|
typeof | Returns the type of a variable or value | typeof 42 | "number" |
instanceof | Tests if an object is an instance of a class | obj instanceof Date | true /false |
constructor | Returns the constructor function of an instance | arr.constructor === Array | true /false |
Practical Example
Let’s combine these type operators in a practical example:
let num = 10;
let str = "JavaScript";
let arr = [1, 2, 3];
let obj = { key: "value" };
let date = new Date();
let func = function() { return "Hello!"; };
console.log(typeof num); // Output: "number"
console.log(str.constructor === String); // Output: true
console.log(arr instanceof Array); // Output: true
console.log(obj instanceof Object); // Output: true
console.log(date instanceof Date); // Output: true
console.log(func instanceof Function); // Output: true
Conclusion
In conclusion, JavaScript operators are essential tools for any developer working with this versatile language. They allow you to manipulate data, make comparisons, control the flow of your program, and perform a wide range of operations. By mastering arithmetic, assignment, comparison, logical, bitwise, conditional (ternary), string, and type operators, you can write more powerful and efficient JavaScript code.
This comprehensive guide has provided you with the foundational knowledge and practical examples needed to understand and utilize these operators effectively. As you continue your journey in JavaScript programming, remember to practice using these operators in different scenarios to reinforce your understanding and improve your coding skills.
Happy coding, and don’t hesitate to reach out if you have any questions or need further clarification!
Get up to 85% off
Build your project using Managed VPS & Dedicated hosting and decrease your workload.
You'll get seamless migration, exceptional savings, 24/7 technical support, HIPAA compliant, PCI compliant, Multi-Level DDoS protection, 30-day money-back guarantee and more with Liquid Web.
Affiliate Disclosure: Affiliate links are available here. If you click through the affiliate links and buy something, we may get a small commission, and you'll not be charged extra.