Mastering javascript one liner: A Comprehensive Guide
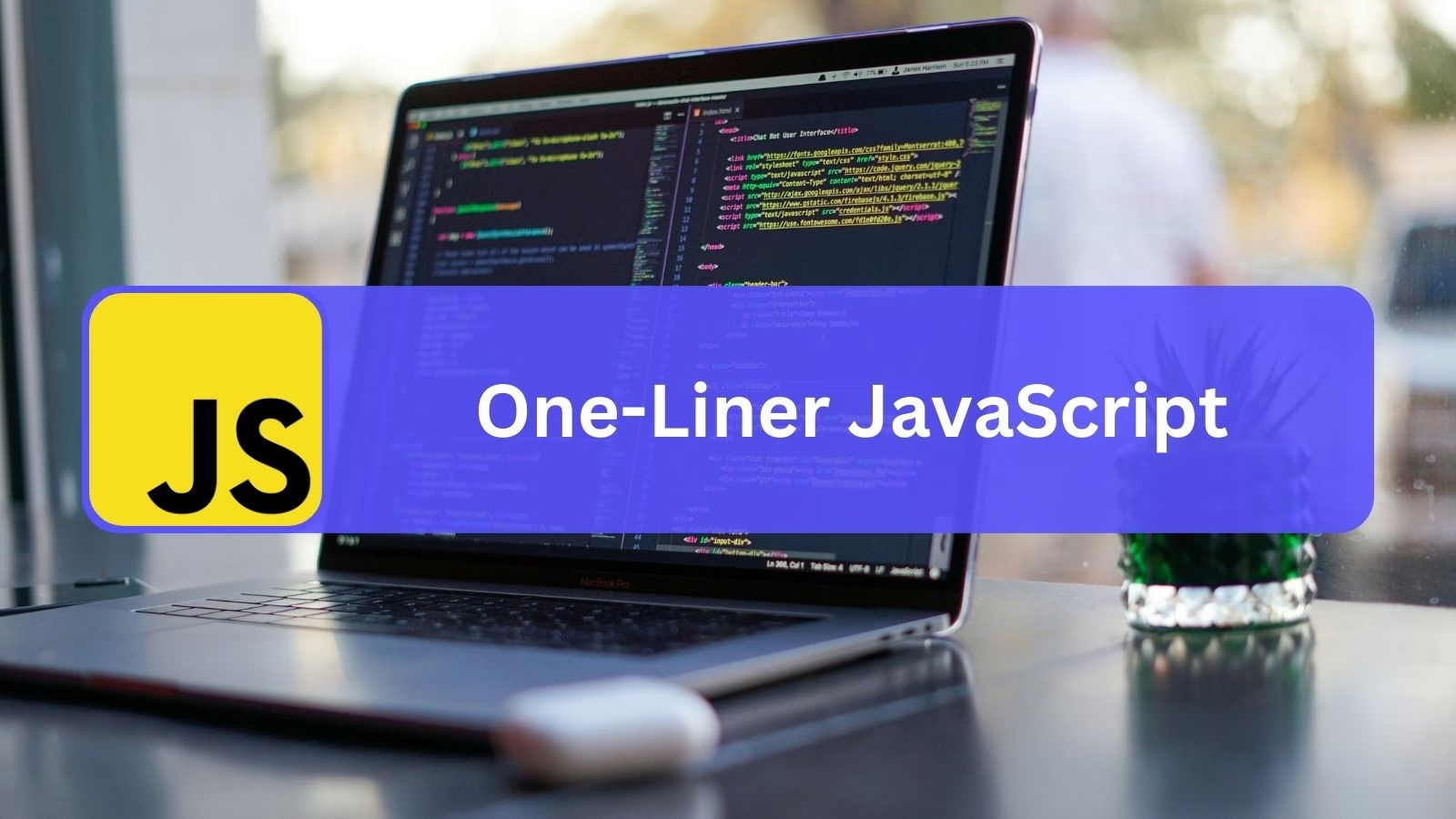
This post was generated with AI and then edited by us.
JavaScript is known for its flexibility and power, allowing developers to write elegant and concise code. One fascinating aspect of JavaScript is the ability to condense complex logic into single lines of code, known as one-liners. These one-liners can make your code more readable and efficient, and mastering them is a skill that can set you apart as a developer.
In this guide, we will explore the art of writing JavaScript one liners. We’ll cover various use cases, from array operations to string manipulations, and provide tips to help you write clean and efficient code. Whether you’re a beginner looking to enhance your coding skills or an experienced developer seeking new techniques, this guide has something for everyone. Let’s dive in and discover the power of JavaScript one-liners!
What is JavaScript one liner?
One-liners in JavaScript are single-line expressions or statements that accomplish a specific task or logic. They are a way to write concise, efficient, and often elegant code that can perform complex operations in just one line. One-liners leverage JavaScript’s powerful functions, operators, and syntax to achieve this.
Here are some simple examples of one-liners in JavaScript, along with explanations:
- Sum of an array:
Explanation: This one-liner uses the reduce
method to sum all the elements in an array. The reduce
method iterates through each element of the array, accumulating the sum in the acc
(accumulator) variable, starting with an initial value of 0
.
const sum = arr => arr.reduce((acc, val) => acc + val, 0);
- Finding the maximum value in an array:
const max = arr => Math.max(...arr);
Explanation: This one-liner uses the Math.max
function along with the spread operator (...
). The spread operator expands the array into individual arguments, which are then passed to Math.max
to find the largest value.
- Reversing a string:
const reverseString = str => str.split('').reverse().join('');
Explanation: This one-liner reverses a string by first splitting it into an array of characters using split('')
, then reversing the array with reverse()
, and finally joining the characters back into a string with join('')
.
- Checking if a number is even or odd:
const isEven = num => num % 2 === 0 ? 'Even' : 'Odd';
Explanation: This one-liner uses the modulo operator (%
) to check if a number is even or odd. If the number divided by 2 has a remainder of 0, it is even; otherwise, it is odd. The ternary operator (? :
) is used to return the appropriate string.
- Mapping an array of objects to an array of values:
const names = users.map(user => user.name);
Explanation: This one-liner uses the map
method to create a new array containing only the names of the users. The map
method iterates through each object in the users
array and extracts the name
property from each object, returning a new array of names.
Why Use JavaScript one liners?
Using one-liners in JavaScript offers several benefits that can significantly enhance your coding experience and improve the overall quality of your code. Here are some key advantages:
1. Readability
Concise code improves readability by eliminating unnecessary boilerplate and focusing on the core logic. One-liners can make your code more straightforward and easier to understand. When used appropriately, they reduce visual clutter and make it clear what the code is doing at a glance.
Example:
const sum = arr => arr.reduce((acc, val) => acc + val, 0);
Explanation: This one-liner uses the reduce
method to sum all the elements in an array. The reduce
method iterates through each element of the array, accumulating the sum in the acc
(accumulator) variable, starting with an initial value of 0
.
2. Efficiency
Fewer lines of code can lead to more efficient performance, especially in cases where the overhead of function calls and extra variables is minimized. One-liners leverage built-in functions and operators, which are optimized for performance.
Example:
const max = arr => Math.max(...arr);
Explanation: This one-liner uses the Math.max
function along with the spread operator (...
). The spread operator expands the array into individual arguments, which are then passed to Math.max
to find the largest value.
3. Elegance
One-liners showcase a deep understanding of JavaScript and its capabilities. They often require a good grasp of the language’s features and idiomatic expressions, which can demonstrate your proficiency as a developer. Writing elegant one-liners can be satisfying and can make your codebase more maintainable.
Example:
const reverseString = str => str.split('').reverse().join('');
Explanation: This single line of code uses array methods to achieve a common task, highlighting the elegance and power of JavaScript.
4. Conciseness
One-liners reduce the amount of code you need to write and maintain, which can save time and reduce the likelihood of errors. They allow you to express your intent clearly and succinctly.
Example:
const isEven = num => num % 2 === 0 ? 'Even' : 'Odd';
Explanation: This one-liner uses the modulo operator (%
) to check if a number is even or odd. If the number divided by 2 has a remainder of 0, it is even; otherwise, it is odd. The ternary operator (? :
) is used to return the appropriate string.
5. Functional Programming
One-liners often leverage functional programming concepts, which can lead to more predictable and testable code. Functional programming emphasizes the use of pure functions and avoids side effects, making your code easier to reason about.
Example:
const names = users.map(user => user.name);
Explanation: This one-liner uses the map
method to create a new array containing only the names of the users. The map
method iterates through each object in the users
array and extracts the name
property from each object, returning a new array of names.
Common Use Cases of One-Liners in JavaScript
One-liners in JavaScript can significantly enhance the efficiency and readability of your code. Here’s a comprehensive look at their common use cases, along with explanations and examples:
1. Array Operations
Summing an Array:
const sum = arr => arr.reduce((acc, val) => acc + val, 0);
Explanation: This one-liner uses the reduce
method to iterate over the elements of an array, accumulating their sum in the acc
(accumulator) variable. It starts with an initial value of 0
and adds each value (val
) in the array to the accumulator.
Finding the Maximum Value in an Array:
const max = arr => Math.max(...arr);
Explanation: This one-liner employs the spread operator (...
) to expand the array elements into individual arguments for the Math.max
function, which then returns the largest value.
2. String Manipulation
Reversing a String:
const reverseString = str => str.split('').reverse().join('');
Explanation: This one-liner splits the string into an array of characters, reverses the array, and then joins the characters back into a string, effectively reversing the original string.
Checking for Palindromes:
const isPalindrome = str => {
const sanitizedStr = str.toLowerCase().replace(/[\W_]/g, '');
return sanitizedStr === sanitizedStr.split('').reverse().join('');
};
Explanation: This one-liner first sanitizes the string by converting it to lowercase and removing non-alphanumeric characters. It then checks if the sanitized string is the same as its reverse, determining if it is a palindrome.
3. Conditionals
Using the Ternary Operator for Conditionals:
const isEven = num => num % 2 === 0 ? 'Even' : 'Odd';
Explanation: This one-liner uses the ternary operator to check if a number is even or odd. If the number modulo 2 equals 0, it returns ‘Even’; otherwise, it returns ‘Odd’.
Short-Circuit Evaluation:
const getDefault = (value, defaultValue) => value || defaultValue;
Explanation: This one-liner uses short-circuit evaluation to return a default value if the given value is falsy. If the value is truthy, it returns the value itself; otherwise, it returns the provided default value.
4. Data Transformation
Mapping Arrays:
const names = users.map(user => user.name);
Explanation: This one-liner uses the map
method to iterate over an array of objects (users
), extracting the name
property from each object and returning a new array of names.
Filtering Arrays:
const adults = users.filter(user => user.age >= 18);
Explanation: This one-liner uses the filter
method to create a new array containing only the users who are 18 years old or older. It iterates through the users
array and includes only those that meet the age condition.
5. Functional Programming
Function Composition:
const compose = (f, g) => x => f(g(x));
Explanation: This one-liner defines a compose
function that takes two functions (f
and g
) and returns a new function. The new function, when called with an argument x
, applies g
to x
and then applies f
to the result of g(x)
.
Currying Functions:
const add = a => b => a + b;
Explanation: This one-liner defines a curried add
function. The add
function takes a single argument a
and returns another function that takes a single argument b
. When the returned function is called with b
, it adds a
and b
.
Writing Effective One-Liners in JavaScript
Crafting effective one-liners in JavaScript is an art that balances conciseness with clarity. These compact expressions can streamline your code, making it more readable and efficient. This comprehensive guide will delve into the principles of writing effective one-liners, leveraging modern JavaScript features, and techniques for refactoring existing code into one-liners.
Clarity Over Conciseness
While one-liners can make your code concise, clarity should always be the top priority. Code that’s too compact can become difficult to understand and maintain. Here are some tips for maintaining clarity:
- Descriptive Naming: Use clear and descriptive variable and function names that convey their purpose.
- Readable Expressions: Break down complex expressions if they compromise readability, even if it means writing a few more lines.
- Commenting: Add comments to explain the purpose and logic of one-liners, especially if they perform intricate operations.
Example:
// Less clear
const p = a => a.map(b => b + 2).filter(c => c > 5);
// More clear with descriptive names and comments
const processArray = arr =>
// Add 2 to each element, then filter out elements <= 5
arr.map(element => element + 2).filter(element => element > 5);
Using Modern JavaScript Features
Modern JavaScript (ES6 and later) introduces features that can help you write effective one-liners. Some of these features include:
- Arrow Functions: Provide a shorter syntax for writing functions.
- Destructuring: Allows extracting values from arrays or objects into distinct variables.
- Spread Operator: Enables expanding elements of an iterable (like an array) into individual elements.
- Template Literals: Offer a convenient way to work with strings and embed expressions.
Examples:
Arrow Functions:
const double = n => n * 2;
Explanation: Arrow functions provide a concise syntax for writing functions, eliminating the need for the function
keyword and braces for simple expressions.
Destructuring:
const [first, second] = [10, 20];
Explanation: Destructuring allows you to unpack values from arrays or properties from objects into distinct variables, making the code more readable and expressive.
Spread Operator:
const max = Math.max(...[1, 2, 3]);
Explanation: The spread operator (...
) expands the elements of an array into individual arguments, enabling more concise and readable code.
Template Literals:
const greet = name => `Hello, ${name}!`;
Explanation: Template literals provide an easy way to create strings with embedded expressions, improving readability and reducing syntax errors.
Refactoring Code
Refactoring existing code into one-liners can improve readability and efficiency. Here are some techniques:
- Identify Patterns: Look for repetitive code patterns that can be condensed.
- Use Built-in Methods: Replace loops and multiple lines of code with built-in array and object methods.
- Simplify Conditionals: Use ternary operators for simple conditional logic.
Example:
Original code:
function getNames(users) {
let names = [];
for (let i = 0; i < users.length; i++) {
names.push(users[i].name);
}
return names;
}
Refactored one-liner:
const getNames = users => users.map(user => user.name);
Explanation: This one-liner uses the map
method to iterate over the users
array and extract the name
property from each object, creating a new array of names.
Another example:
Original code:
function isAdult(age) {
if (age >= 18) {
return true;
} else {
return false;
}
}
Refactored one-liner:
const isAdult = age => age >= 18;
Explanation: This one-liner uses a simple comparison and eliminates the need for an if-else
statement, making the code more concise and readable.
Potential Pitfalls of Using One-Liners
While one-liners in JavaScript can be powerful tools, it’s essential to use them wisely to avoid potential pitfalls. Here are some common issues that developers may encounter when using one-liners excessively:
1. Overuse
Dangers of Using One-Liners Excessively:
- Readability: Overusing one-liners can make your code difficult to read and understand, especially for those who are not familiar with the language or the specific techniques used. This can hinder collaboration and maintenance.
- Maintainability: When code is too concise, it can be challenging to modify or extend in the future. Clear and well-structured code is easier to maintain and adapt to new requirements.
Example of Overuse:
// Overly concise and hard to read
const result = arr.reduce((acc, val) => (val % 2 === 0 ? acc.concat(val * 2) : acc), []).sort((a, b) => b - a).slice(0, 5);
Explanation: This one-liner performs multiple operations (filtering, mapping, sorting, slicing) in a single line, making it difficult to understand and maintain.
2. Complexity
How Overly Complex One-Liners Can Hinder Readability:
- Cognitive Load: Complex one-liners increase the cognitive load on the reader, making it harder to grasp the code’s logic quickly. This can lead to misunderstandings and errors.
- Debugging Challenges: Complex one-liners can be challenging to debug, as it is not always clear which part of the expression is causing an issue.
Example of Complexity:
// Complex and hard to debug
const process = str => str.split('').reduce((acc, char) => (char === char.toUpperCase() ? acc + char.toLowerCase() : acc + char.toUpperCase()), '');
Explanation: This one-liner attempts to toggle the case of each character in a string, but the complexity makes it difficult to read and debug.
3. Debugging
Challenges in Debugging One-Liners:
- Limited Breakpoints: In many debugging tools, setting breakpoints within one-liners is not possible, making it harder to inspect intermediate values and identify issues.
- Error Tracing: When an error occurs in a one-liner, it can be difficult to trace the source of the problem, especially if multiple operations are chained together.
Example of Debugging Issue:
// Debugging challenge with chained methods
const transform = arr => arr.map(n => n * 2).filter(n => n > 10).reduce((acc, n) => acc + n, 0);
Explanation: If an error occurs, it is challenging to determine whether it happened during mapping, filtering, or reducing.
Best Practices for Writing One-Liners in JavaScript
Crafting effective and maintainable one-liners requires a balance between conciseness and clarity. Here are some best practices to help you write better one-liners and decide when to use them:
Guidelines for Writing Maintainable One-Liners
- Descriptive Naming: Use clear and descriptive variable and function names that convey their purpose. Avoid using single-letter names unless they are commonly understood (e.g.,
i
for index).
// Less descriptive
const s = arr => arr.reduce((a, b) => a + b, 0);
// More descriptive
const sumArray = array => array.reduce((accumulator, currentValue) => accumulator + currentValue, 0);
- Break Down Complex Logic: If the logic is too complex for a single line, break it down into multiple lines for better readability.
// Complex one-liner
const process = str => str.split('').reduce((acc, char) => (char === char.toUpperCase() ? acc + char.toLowerCase() : acc + char.toUpperCase()), '');
// More readable
const toggleCase = char => char === char.toUpperCase() ? char.toLowerCase() : char.toUpperCase();
const process = str => str.split('').reduce((acc, char) => acc + toggleCase(char), '');
- Use Comments: Add comments to explain the purpose and logic of one-liners, especially if they perform intricate operations.
// Toggle the case of each character in a string
const toggleCase = char => char === char.toUpperCase() ? char.toLowerCase() : char.toUpperCase();
Balancing Conciseness with Clarity
- Prioritize Readability: Always ensure that your one-liners are clear and understandable. If a one-liner becomes too convoluted, it’s better to break it down into multiple lines.
// Clear and concise
const isEven = num => num % 2 === 0 ? 'Even' : 'Odd';
- Leverage Modern JavaScript Features: Use ES6+ features like arrow functions, destructuring, and the spread operator to write clean and concise code.
// Using destructuring and spread operator
const [first, ...rest] = [1, 2, 3, 4];
const max = Math.max(...rest);
- Refactor When Necessary: If you find that a one-liner is too complex, refactor it into multiple lines to improve clarity.
// Complex one-liner
const transform = arr => arr.map(n => n * 2).filter(n => n > 10).reduce((acc, n) => acc + n, 0);
// Refactored for clarity
const doubled = arr.map(n => n * 2);
const filtered = doubled.filter(n => n > 10);
const sum = filtered.reduce((acc, n) => acc + n, 0);
When to Use and When to Avoid One-Liners
Use One-Liners When:
- The logic is simple and easily understood.
- The one-liner improves readability and maintainability.
- It leverages built-in methods and modern features effectively.
Avoid One-Liners When:
- The logic is complex and hard to follow.
- It compromises readability and makes the code difficult to understand.
- Debugging becomes challenging due to the compact nature of the code.
Advanced Topics: One-Liners in JavaScript
As you delve deeper into JavaScript, you encounter advanced concepts that can also be encapsulated in elegant one-liners. Here are some advanced topics where one-liners can be particularly useful:
One-Liners in Asynchronous Programming
Asynchronous programming in JavaScript allows you to perform tasks without blocking the main thread. One-liners can make asynchronous code more readable and concise.
Example:
// Fetching data from an API
const fetchData = async url => (await fetch(url)).json();
Explanation:
async
keyword allows the function to useawait
inside.await fetch(url)
waits for thefetch
call to complete..json()
parses the response as JSON.- The function returns the parsed JSON data.
One-Liners with Promises and async/await
Promises and async/await are essential for handling asynchronous operations in JavaScript. One-liners can simplify the handling of Promises.
Example with Promises:
// Chaining Promises
const getUserData = userId => fetch(`/api/users/${userId}`).then(res => res.json());
Explanation:
fetch
makes an HTTP request to the given URL..then(res => res.json())
chains a Promise to parse the response as JSON.
Example with async/await:
// Using async/await in one-liner
const getUserData = async userId => (await fetch(`/api/users/${userId}`)).json();
Explanation:
async
keyword allows the function to useawait
.await fetch(
/api/users/${userId})
waits for thefetch
call to complete..json()
parses the response as JSON.
One-Liners in Functional Programming Libraries (e.g., Lodash)
Lodash is a popular utility library that provides many useful functions for functional programming. One-liners can leverage Lodash to write concise and expressive code.
Example with Lodash:
// Using Lodash to get unique elements
const uniqueElements = arr => _.uniq(arr);
Explanation:
_.uniq(arr)
is a Lodash function that returns a new array with only unique elements from the original array.
Another example with Lodash:
// Using Lodash to flatten an array
const flattenArray = arr => _.flatten(arr);
Explanation:
_.flatten(arr)
is a Lodash function that flattens a nested array into a single array.
Combining Lodash functions:
// Chaining Lodash functions for data processing
const processData = arr => _.uniq(_.flatten(arr)).filter(x => x > 10);
Explanation:
_.flatten(arr)
flattens the nested array._.uniq
removes duplicate elements.filter(x => x > 10)
filters out elements less than or equal to 10.
Conclusion
Mastering one-liners in JavaScript is a skill that comes with practice. The more you experiment with writing one-liners, the more intuitive and natural it becomes. Start with simple tasks and gradually challenge yourself with more complex scenarios. Remember, the goal is to write code that is both concise and clear, ensuring it remains maintainable and understandable.
One-liners can significantly enhance the readability, efficiency, and elegance of your code. By understanding their benefits, potential pitfalls, and best practices, you can harness their power to write cleaner, more maintainable code. Keep practicing, experimenting, and pushing the boundaries of what’s possible with JavaScript one-liners.
Happy coding!
Get up to 85% off
Build your project using Managed VPS & Dedicated hosting and decrease your workload.
You'll get seamless migration, exceptional savings, 24/7 technical support, HIPAA compliant, PCI compliant, Multi-Level DDoS protection, 30-day money-back guarantee and more with Liquid Web.
Affiliate Disclosure: Affiliate links are available here. If you click through the affiliate links and buy something, we may get a small commission, and you'll not be charged extra.