How to Reverse a String in JavaScript: A Comprehensive Guide
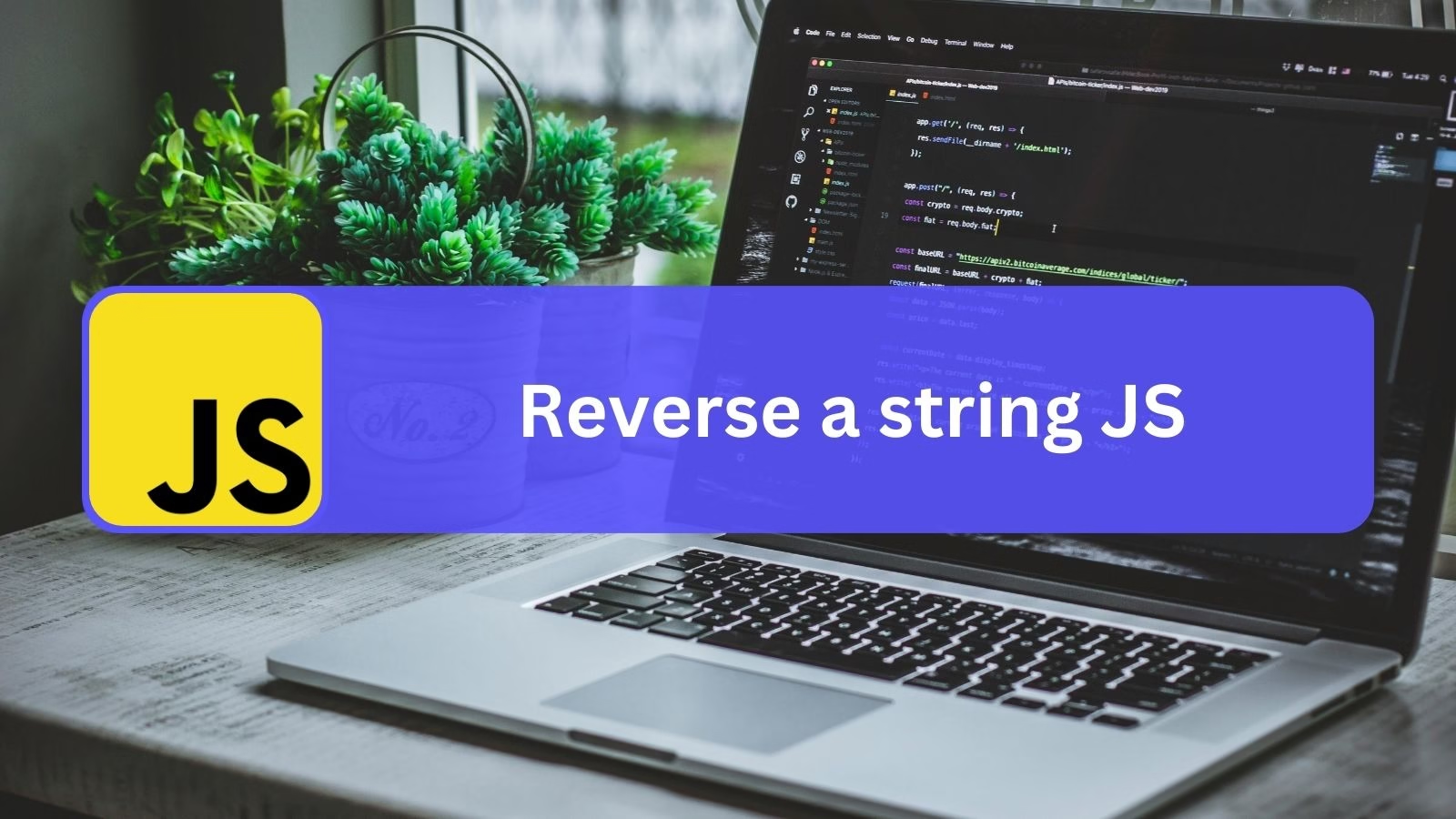
This post was generated with AI and then edited by us.
Strings are fundamental to programming, and sometimes, you need to turn them inside out – literally! String reversal, the process of flipping a string’s characters from first to last, is a surprisingly common task. Whether you’re checking for palindromes, manipulating data, or tackling coding challenges, knowing how to reverse a string in JavaScript is a valuable skill.
This post dives into the various ways you can achieve this, from concise built-in methods to more algorithmic approaches, giving you a comprehensive toolkit for string reversal in JavaScript. Let’s get those strings turning!
What is string reversal in JavaScript?
In JavaScript, string reversal is the process of taking a string (a sequence of characters) and creating a new string where the characters are in the opposite order. So, the first character of the original string becomes the last character of the reversed string, the second character becomes the second-to-last, and so on. It’s like flipping the string end-to-end.
For example, if you have the string “hello”, reversing it would give you “olleh”. JavaScript provides several ways to accomplish this string reversal, which we can explore further if you’d like.
How to Reverse a String in JavaScript?
Reversing a string is a common programming task, and JavaScript offers several ways to achieve this. This blog post will explore different methods, from simple built-in functions to more intricate algorithms, explaining the logic behind each approach and their performance considerations.
Using split()
, reverse()
, and join()
This is the most straightforward and often the most readable approach. It leverages JavaScript’s built-in array methods.
function reverseString(str) {
return str.split("").reverse().join("");
}
console.log(reverseString("hello")); // Output: olleh
console.log(reverseString("JavaScript")); // Output: tpircSavaJ
Here’s how it works
split("")
: This splits the string into an array of individual characters. An empty string as the delimiter ensures each character becomes a separate element in the array.reverse()
: This method reverses the order of the elements within the array.join("")
: This joins the elements of the reversed array back into a string, using an empty string as the separator (resulting in a continuous string).
Using a for
loop (Iterative Approach)
This method iterates through the string from the end to the beginning, building the reversed string character by character.
function reverseString(str) {
let reversedString = "";
for (let i = str.length - 1; i >= 0; i--) {
reversedString += str[i];
}
return reversedString;
}
console.log(reverseString("hello")); // Output: olleh
Here’s the breakdown
let reversedString = "";
: Initializes an empty string to store the reversed characters.for (let i = str.length - 1; i >= 0; i--)
: Afor
loop starts at the last character of the string (str.length - 1
) and iterates backward to the first character (index 0).reversedString += str[i];
: In each iteration, the current character (str[i]
) is appended to thereversedString
.
Using for...of
loop (Modern Iteration)
Similar to the previous approach, but using the more modern for...of
loop for character iteration.
function reverseString(str) {
let reversedString = "";
for (const char of str) {
reversedString = char + reversedString; // Prepend each character
}
return reversedString;
}
console.log(reverseString("hello")); // Output: olleh
The key difference here is reversedString = char + reversedString;
. Instead of appending, we prepend each character to the reversedString
. This effectively reverses the order.
Using reduce()
(Functional Approach)
This method uses the reduce()
function, a powerful tool in functional programming, to reverse the string.
function reverseString(str) {
return str.split("").reduce((reversed, char) => char + reversed, "");
}
console.log(reverseString("hello")); // Output: olleh
Here’s how it works
str.split("")
: Splits the string into an array of characters.reduce((reversed, char) => char + reversed, "")
: Thereduce()
function iterates through the character array.reversed
: The accumulator, which starts as an empty string (“”). It stores the reversed portion of the string so far.char
: The current character being processed.char + reversed
: Prepends the current character to thereversed
string.
Performance Considerations
While all these methods achieve the desired result, their performance can vary slightly, especially with very large strings.
- The
split().reverse().join()
approach is generally efficient and often the most performant for most common use cases. - The iterative
for
loop andfor...of
loop approaches are also quite efficient. - The
reduce()
approach can be slightly less performant than the others in some JavaScript engines, but the difference is often negligible.
Important Note on Performance: Micro-optimizations in string reversal are usually not necessary unless you’re dealing with extremely large strings or performance-critical applications. Focus on writing clear and maintainable code first. If performance becomes a bottleneck, then benchmark the different methods in your specific environment to determine the most efficient approach.
Comparing string reversal methods
Here’s a comparison of the different string reversal approaches in JavaScript.
Method | Description | Readability | Performance (General) | Memory Usage (General) | Functional/Imperative |
---|---|---|---|---|---|
split().reverse().join() | Splits into array, reverses, joins back into string. | High | Good | Moderate | Functional |
for loop (iterative) | Iterates backward, appending characters to a new string. | Medium | Good | Low | Imperative |
for...of loop (iterative) | Iterates using for…of, prepending characters. | Medium | Good | Low | Imperative |
reduce() | Uses reduce() to build the reversed string. | Medium | Can be slightly less | Moderate | Functional |
Explanation of Columns:
- Method: The specific JavaScript code used for the reversal.
- Description: A brief explanation of how the method works.
- Readability: How easy the code is to understand and maintain. “High” means it’s generally considered clear and straightforward. “Medium” means it requires a little more thought to follow the logic.
- Performance (General): A general assessment of performance. “Good” means it’s generally efficient for most use cases. “Can be slightly less” indicates that in some JavaScript engines or with very large strings, it might not be the absolute fastest, though the difference is often negligible. It’s always recommended to benchmark for your specific use case if performance is critical.
- Memory Usage (General): A general assessment of memory usage. “Low” means it generally uses less memory. “Moderate” means it might use a bit more, particularly the
split()
method as it creates an intermediate array. - Functional/Imperative: Indicates the programming paradigm the method leans towards. “Functional” uses higher-order functions like
reduce()
. “Imperative” uses loops and explicit state changes.
Which method to choose?
- For most common use cases,
split().reverse().join()
is often preferred due to its balance of readability and performance. - The iterative approaches (
for
andfor...of
) are good alternatives and offer greater control if you have specific requirements. They are often slightly more memory-efficient. reduce()
provides a concise functional approach, but it might be slightly less performant in some situations.
Conclusion
So, there you have it!
We’ve explored four ways to reverse a string in JavaScript: the concise split().reverse().join()
method, the classic iterative approach using a for
loop, the modern for...of
loop iteration, and the functional reduce()
technique.
Each method offers its own balance of readability, performance, and style. While split().reverse().join()
often takes the crown for simplicity and general efficiency, understanding the iterative and functional alternatives gives you the flexibility to choose the best tool for the job.
Whether you’re working on a palindrome checker, a cryptography project, or just brushing up on your coding skills, mastering these four string reversal methods in JavaScript is a valuable asset.
Now go forth and flip those strings! you prefer using built-in methods, loops, or recursion, understanding these techniques will enhance your coding skills and prepare you for various programming challenges.
Get up to 85% off
Build your project using Managed VPS & Dedicated hosting and decrease your workload.
You'll get seamless migration, exceptional savings, 24/7 technical support, HIPAA compliant, PCI compliant, Multi-Level DDoS protection, 30-day money-back guarantee and more with Liquid Web.
Affiliate Disclosure: Affiliate links are available here. If you click through the affiliate links and buy something, we may get a small commission, and you'll not be charged extra.