How to write a Hello World program in JavaScript
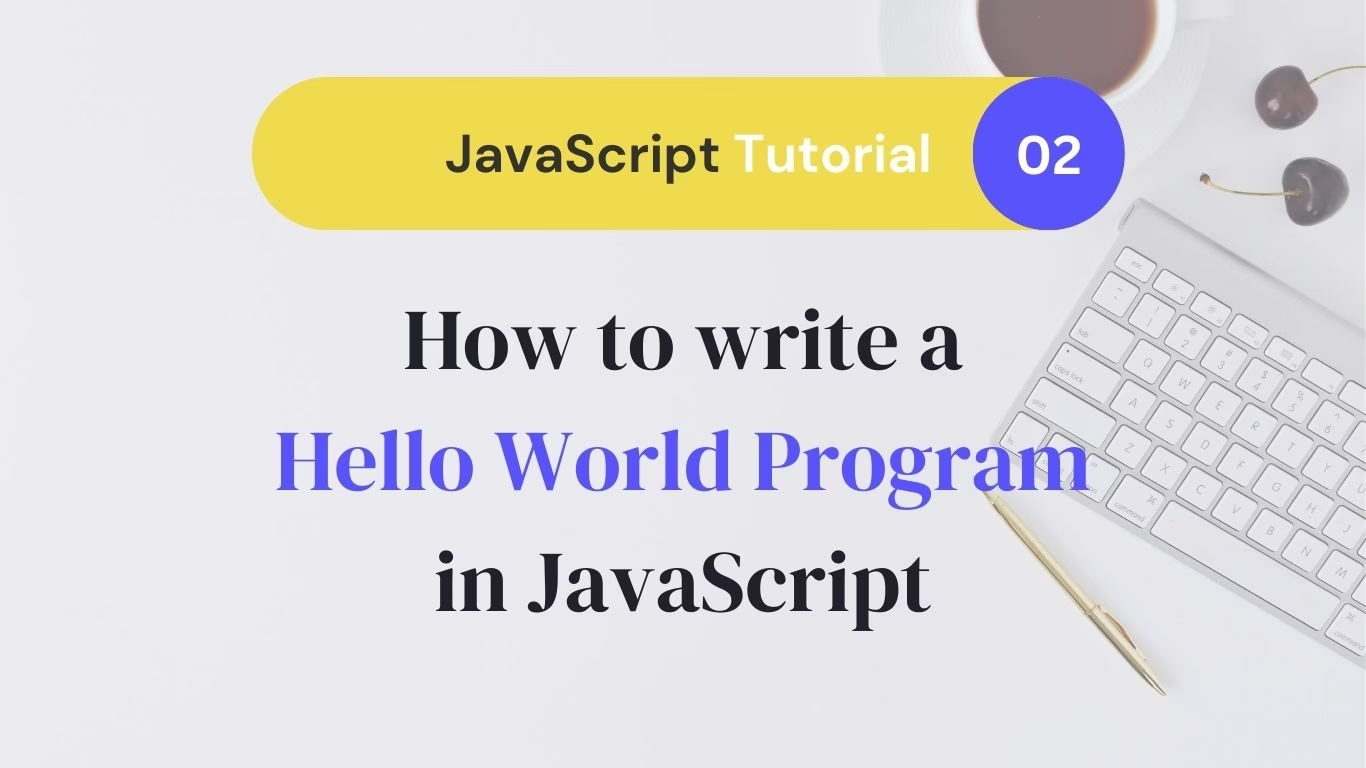
Welcome to the 2nd post of the JavaScript Tutorial Series. This post will show you multiple ways to write a Hello World program in JavaScript.
You’ve stumbled upon this post, which means you’ve chosen to learn JavaScript. We’re glad to have you with us.
Hello World is the first program beginners write when they begin their journey to become developer. Now, let’s get into what is Hello World Program.
What is the Hello World Program?
Hello World is a basic program that displays “Hello World” on the screen.
It is used as a basic code example to show beginners how syntax and structure of a code work in any programming language.
You can’t simply write Hello World, you have to follow rules of syntax and structure to make it work, otherwise, you’ll get an error.
But where to write Hello World code?
Since you’re writing in JavaScript language and JavaScript runs on a web browser by default, you can use your browser’s developer console to write the Hello World program. But that’s not what JavaScript developers use to do programming.
Developers write their code in the proper developer environment. If you want to write a Hello World program in a proper JavaScript developer environment then check the prerequisites below.
Prerequisites for creating Hello World program in JavaScript
To write the Hello World program JavaScript, you need to install a few applications on your computer. These applications are used to create JavaScript developer environment.
- Code Editor (VS Code)
- Runtime environment (Node.js)
- Web Browser with Developer Tools (Google Chrome)
Important: If you need a step-by-step guide to set up your JavaScript development environment, you can visit our post Setting up VS Code for JavaScript Development Environment. There we have explained how to set up VS Code with node.js and a web browser.
But why do you need a Code Editor, Runtime environment and a web browser?
If you want to do coding you need to have a place where you can write your code. A code editor is a type of software in which developers write their code and edit it with the help of advanced developer tools.
A runtime environment helps JavaScript run on a local computer. Since JavaScript was created for running on the web, it cannot run on a computer on its own. So you need to install it on your PC for running JavaScript code.
You need a modern web browser because every modern web browser comes with a developer tool. Moreover, every modern web browser supports JavaScript by default. You don’t need to install a browser separately though. Every Operating System (Windows, MacOS, Linux) comes with a modern web browser.
If you have already finished your set-up process, you can proceed to write the Hello World program.
How to write a Hello World program in JavaScript?
Hello World program is simply a piece of code that displays Hello World. There are multiple ways to do the same tasks. We’ll show you 3 ways to write a Hello World program in JavaScript.
- You can use your web browser’s developer console to write the Hello World program.
- You can use your code editor to write a Hello World program and display it in the output tab.
- You can JavaScript to HTML to create a Hello World program and open it in the browser.
Let’s get into each method to display Hello World.
Write the Hello World program in your browser
Every Operating System comes with a modern web browser. You don’t need to download it separately. Windows comes with Microsoft Edge, MacOS comes with Safari, and Linux comes with Firefox. Many people also prefer to use Google Chrome.
All of these web browsers are modern and come with developer tools so you can use any web browser you like.
Since Google Chrome is the most popular with over 67% usage rate according to web browser market share, we’ll use Google Chrome to demonstrate how to write a Hello World program.
First, open the Google Chrome web browser and right-click on your mouse. Then go to Inspect.
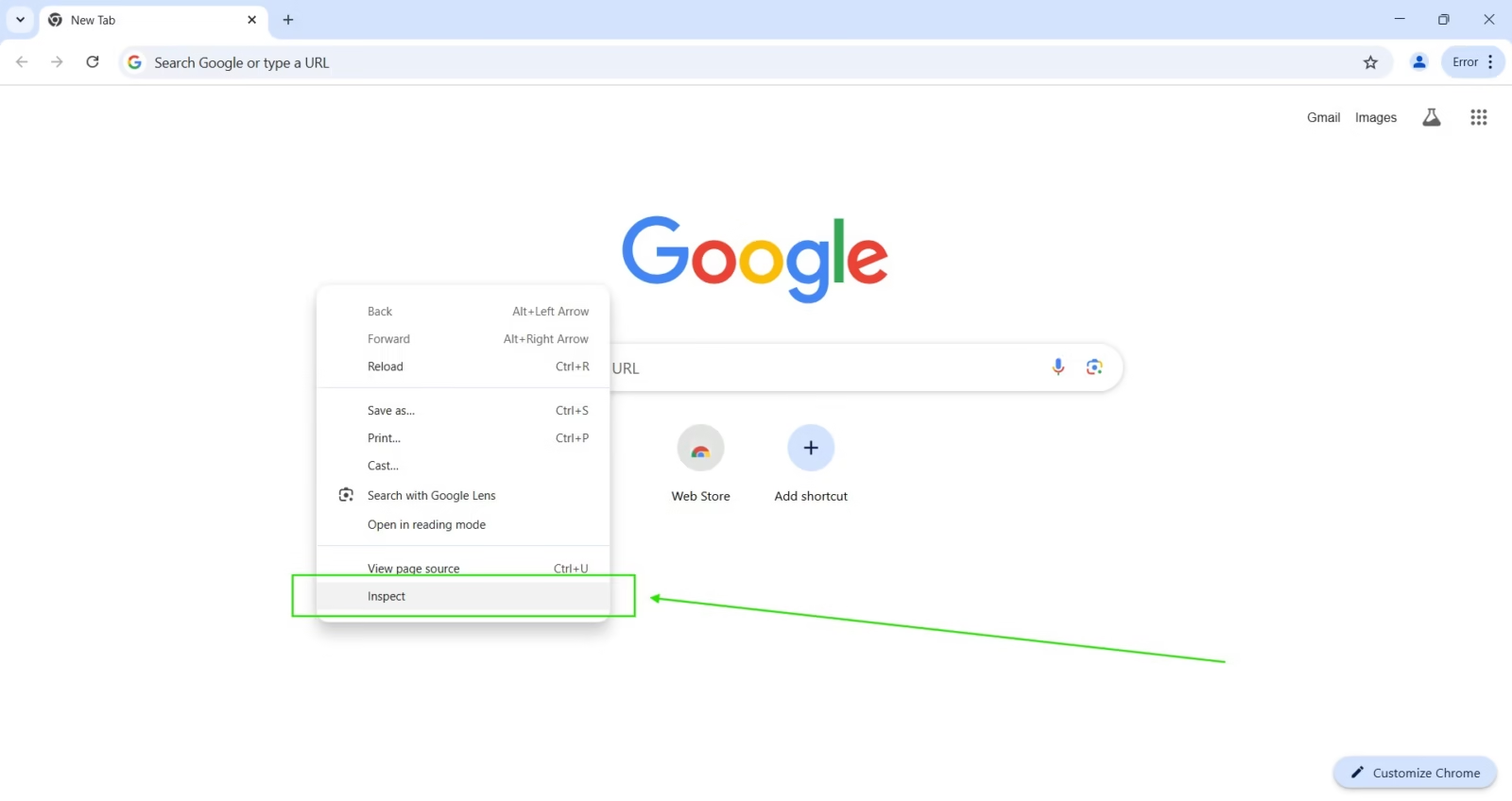
After clicking Inspect Chrome DevTools will open. This is Google Chrome’s developer tool. Now go to the Console tab as shown below.
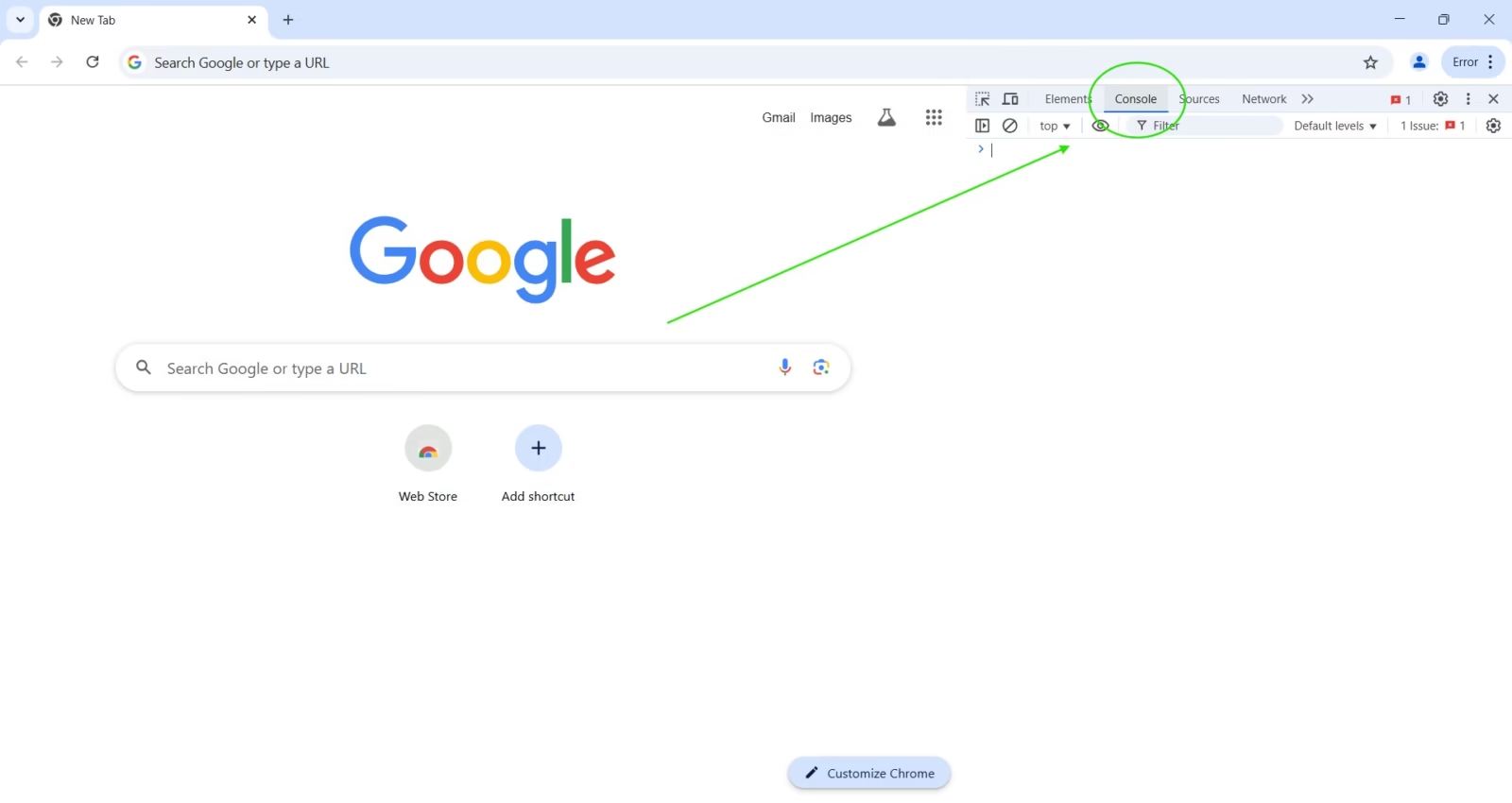
Now type your Hello World program in the Console tab and press Enter. Hello World program has only 1 line of code. You have to type the code exactly as shown below.
console.log("Hello World!");
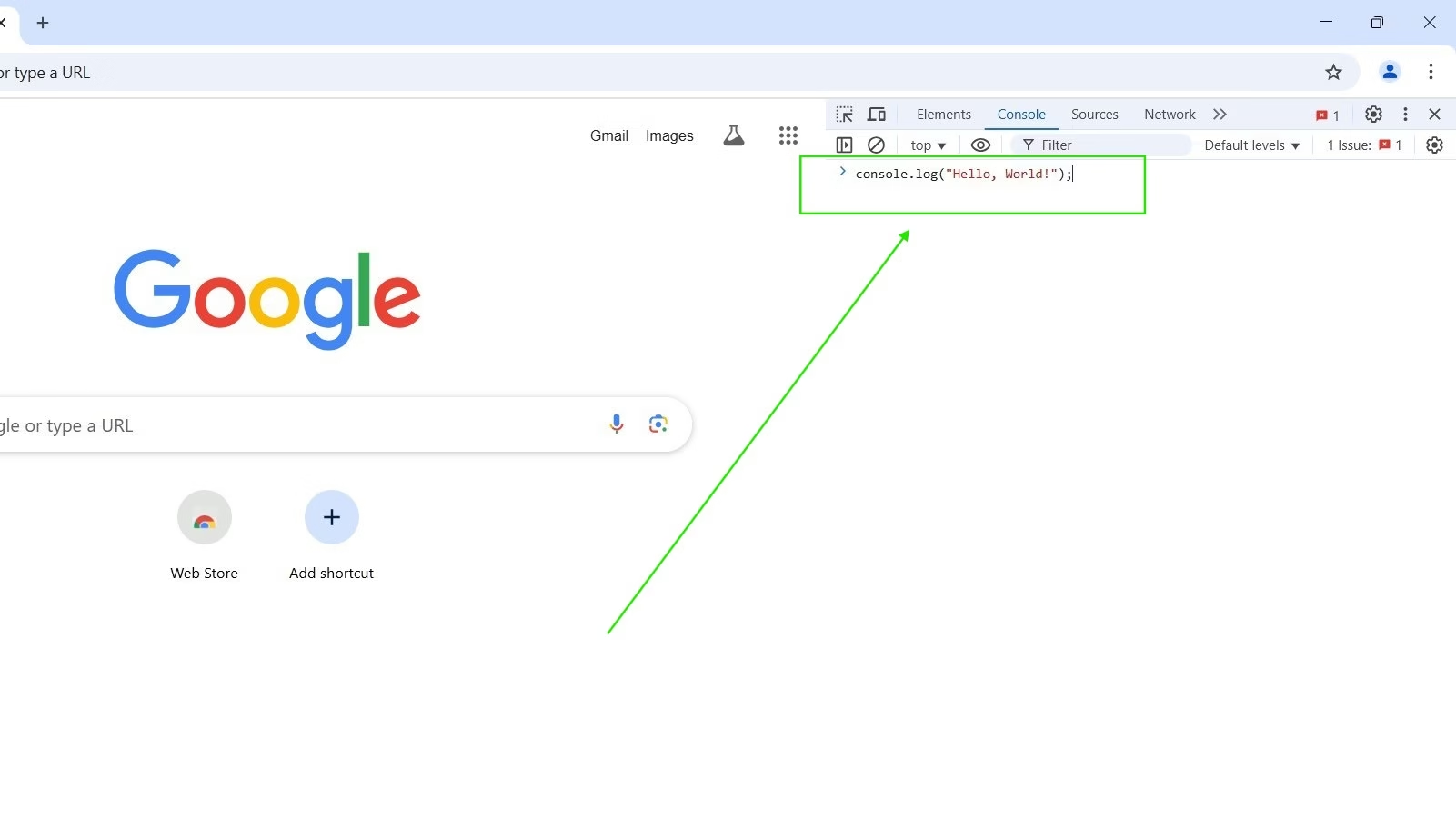
After you type the Hello World code and press Enter, the Output of your code will be visible just below your code.
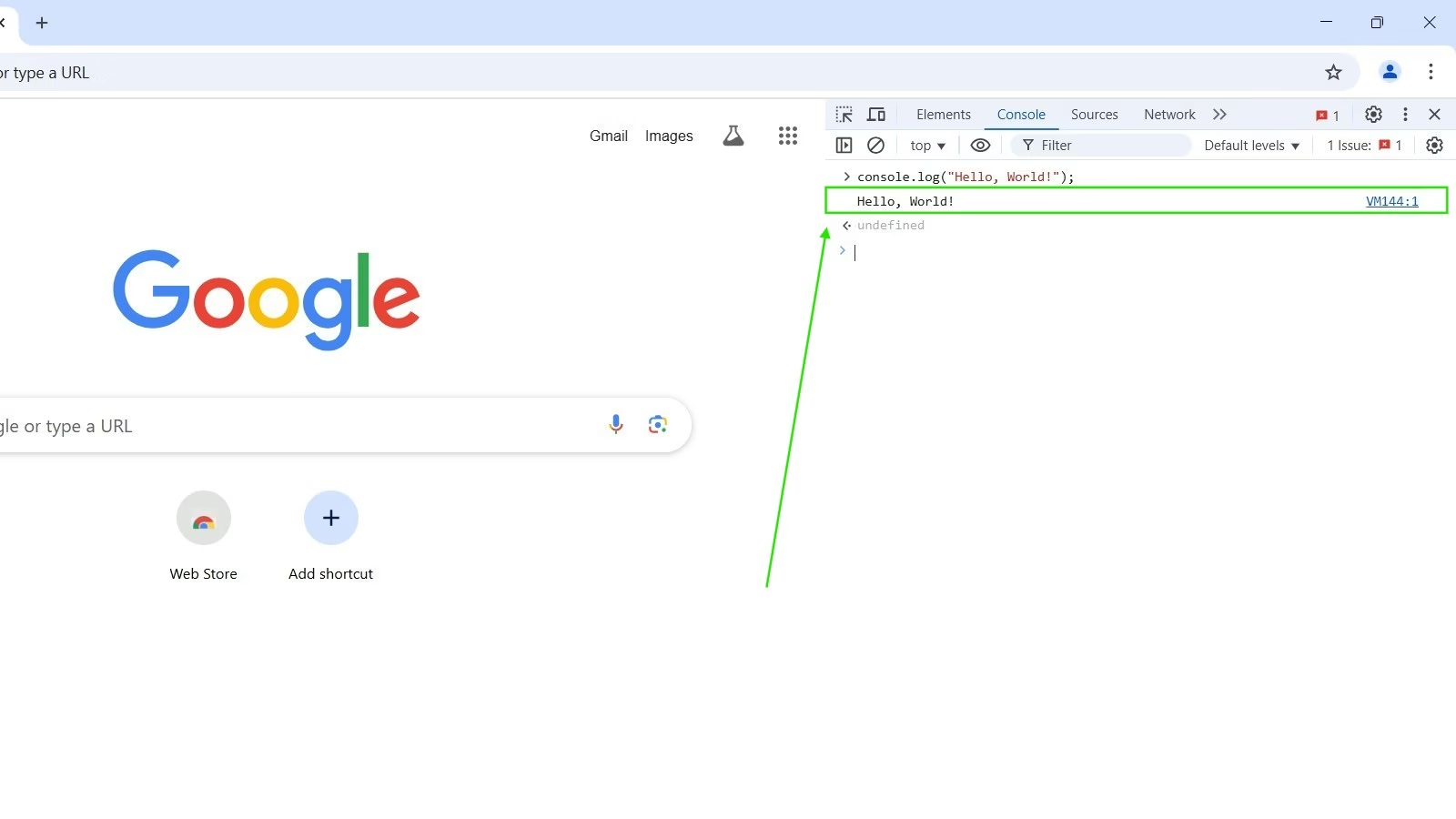
Great, you have just written your first JavaScript program. It doesn’t seem much right? Let’s do the same in your Code editor.
Write Hello World program in Code Editor
As mentioned before, a Code editor is an application where developers write their code and edit it with advanced developer tools. Code editors have built-in features for creating different types of environments for different types of projects.
So for writing JavaScript code, you have to install a few things along with Code Editor and set up your developer environment properly.
Here is everything you need to do:
- You have to install VS Code. It is the most popular Code Editor.
- You have to install Node.js (Runtime Environment) on your PC. JavaScript was built to work on the web, so we need a runtime environment to work on Computer.
- You have to install Code Runner Extention in VS Code. To run JavaScript code and show output you need it.
For the full set-up guide visit our post Setting up VS Code for JavaScript Development Environment and come back to this post after you finish your set-up process.
Now, let’s get into how to write the Hello World program in VS Code.
Open VS Code and go to Explorer.
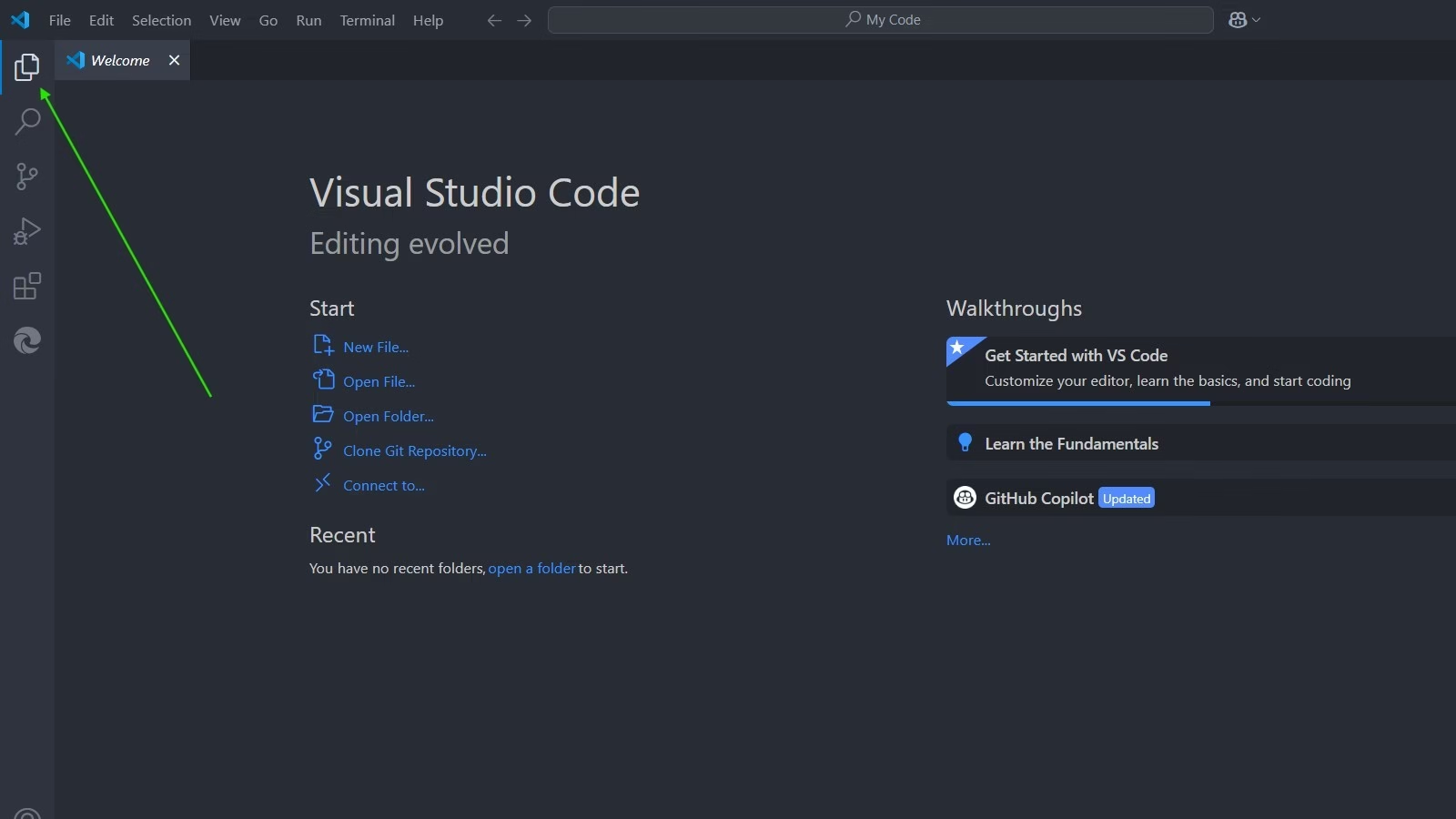
After opening Explorer, click on the File icon to create a new file.
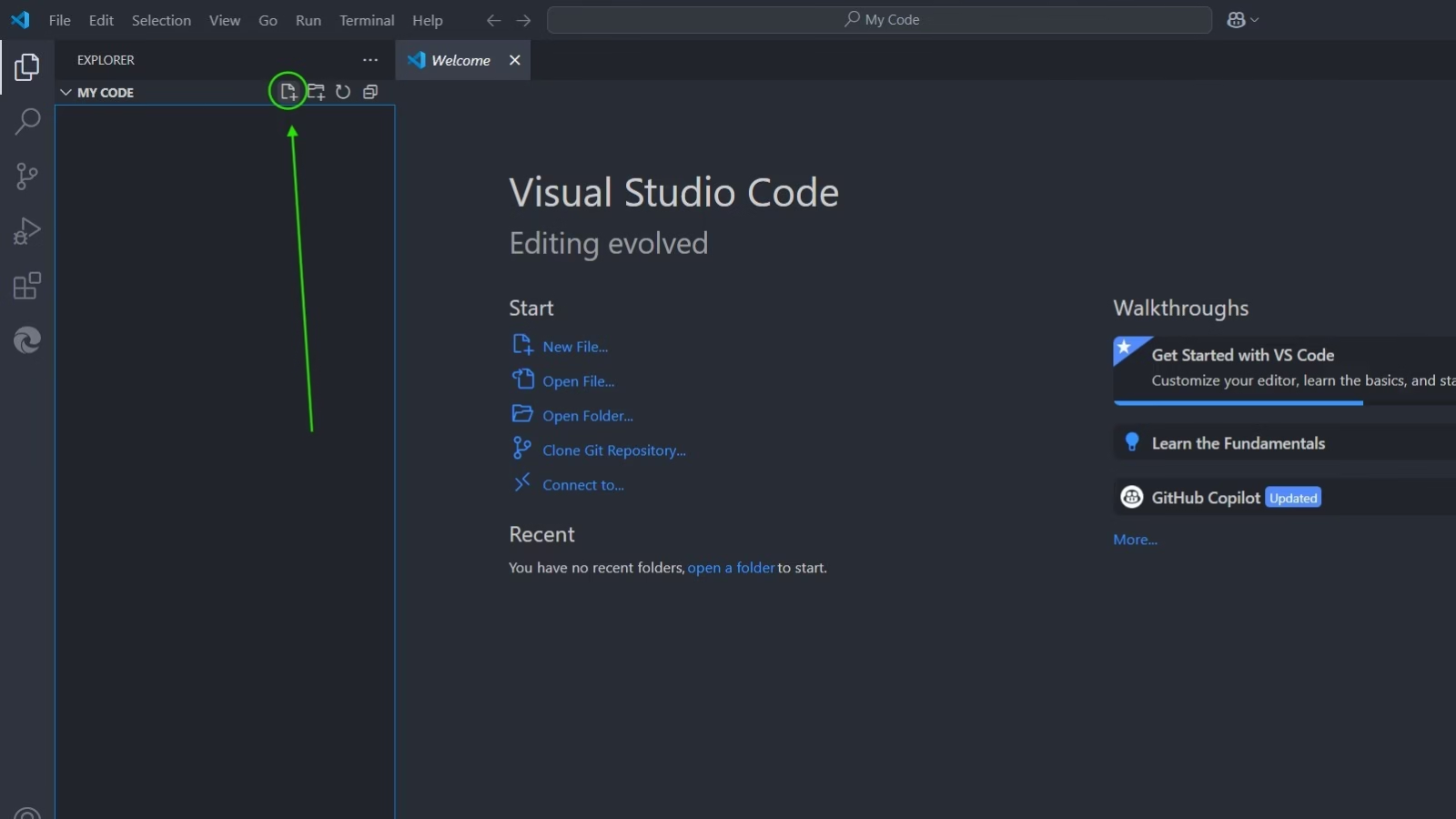
Now name your new file script.js
. Note: All JavaScript file has .js
Extension.
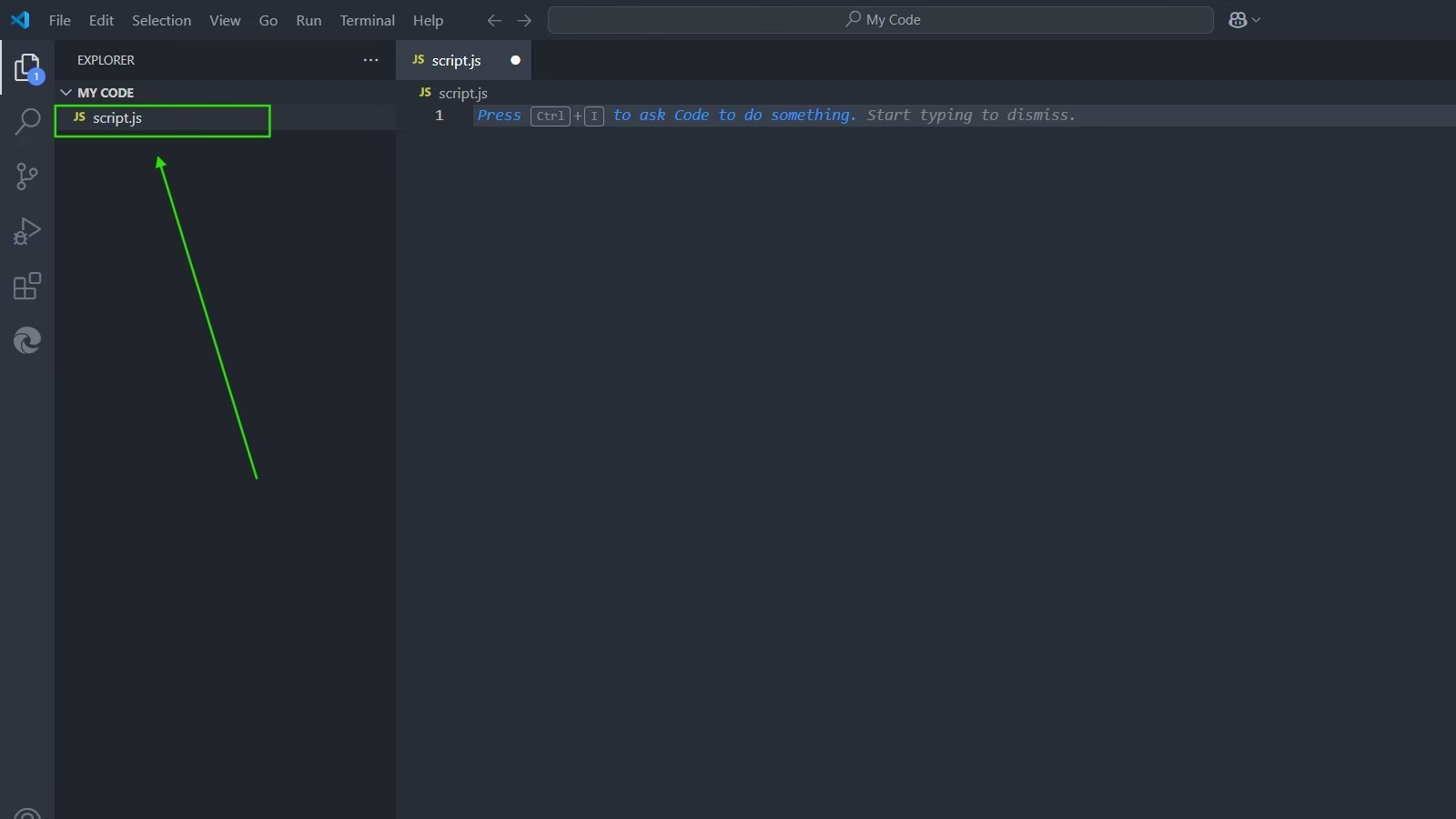
Now write the Hello World program in your JavaScript file. A code example is given below, type the code exactly as shown in the example. Then Save the file. To save in Windows use Ctrl + S. To save in Mac use Cmd + S. You can also use File > Save from the upper left corner of your screen.
console.log("Hello World!");
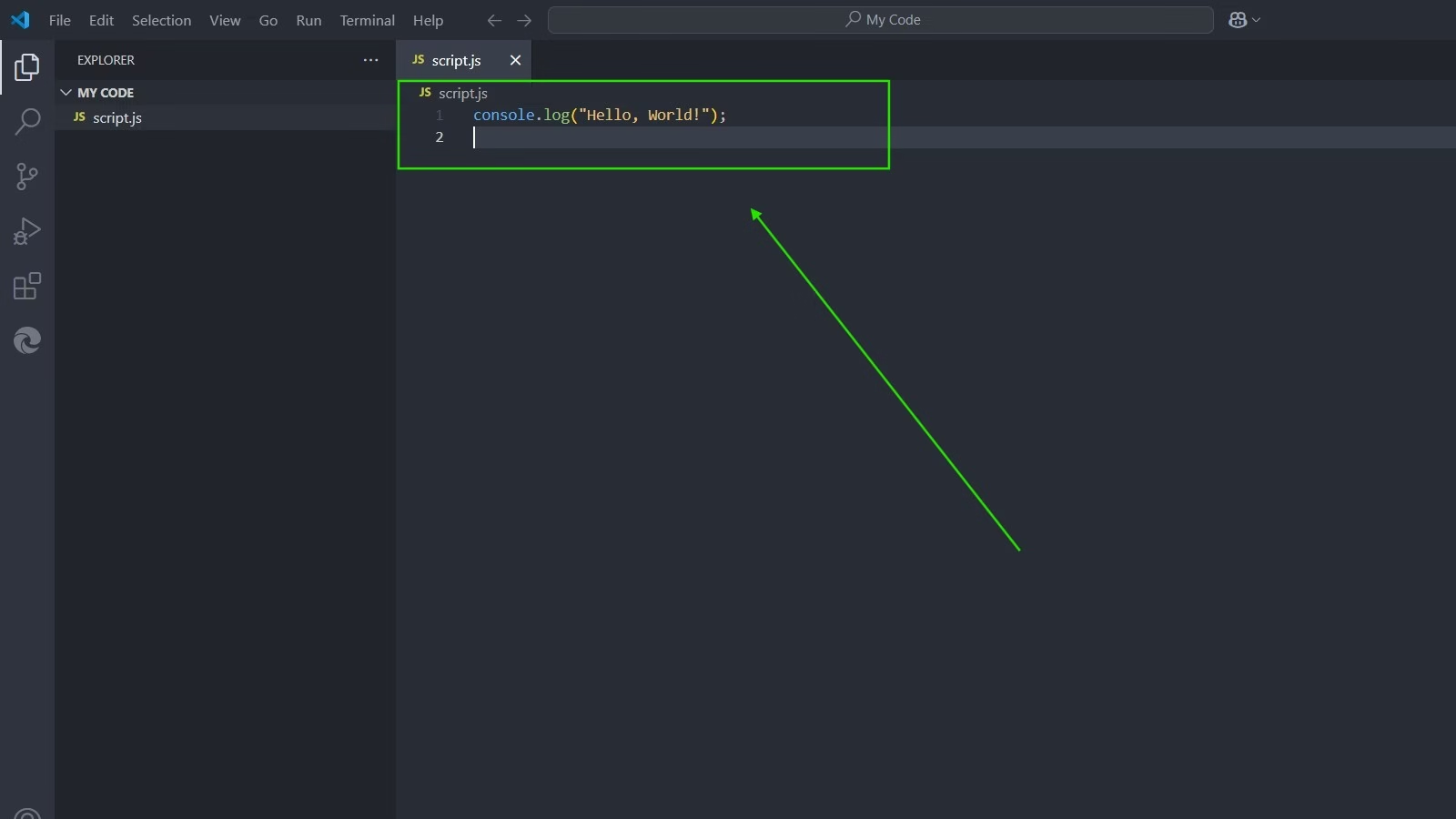
After saving your code, click on the play button in the upper left corner. This will open the Output tab. If you’re not seeing the Play button then you may have to install Node.js again along with Code Runner Extension.
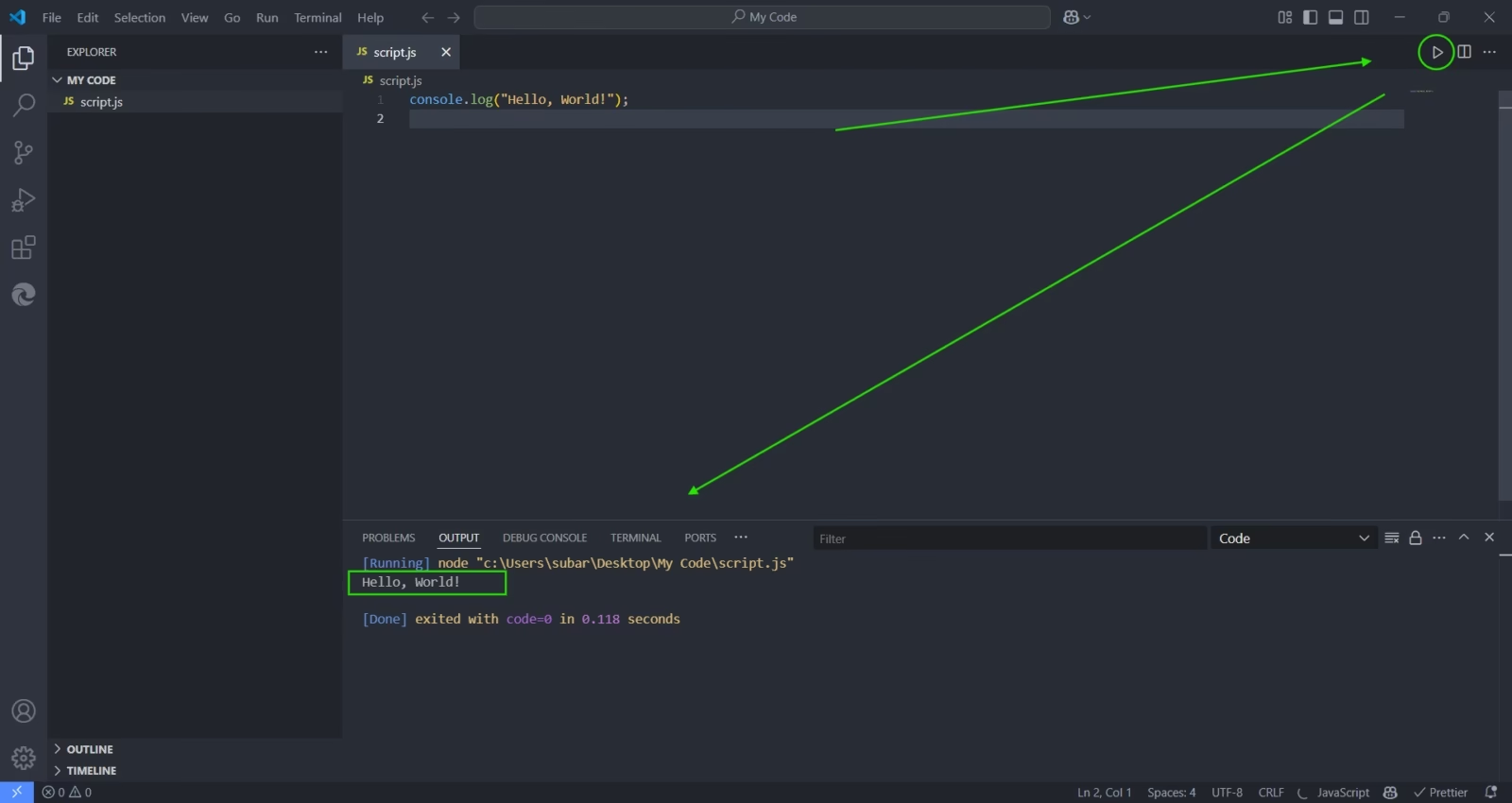
After clicking the play button, you should be able to see the Hello World output.
Congrats! You have correctly written the Hello World Program in JavaScript language.
Now, let’s get into the last method.
Link JavaScript to HTML to create a Hello World program (External JavaScript)
This method for creating a Hello World program may become overwhelming for some people. This is not hard but it is a bit lengthy method.
Here we’ll create separate files for HTML and JavaScript code. Then we will create an HTML boilerplate which will also include code to connect JavaScript with the HTML file. Then we’ll open the HTML file with the web browser to see the result of our code.
First, go to Explorer and click on the File icon to create a new file. Name the new file index.html
.
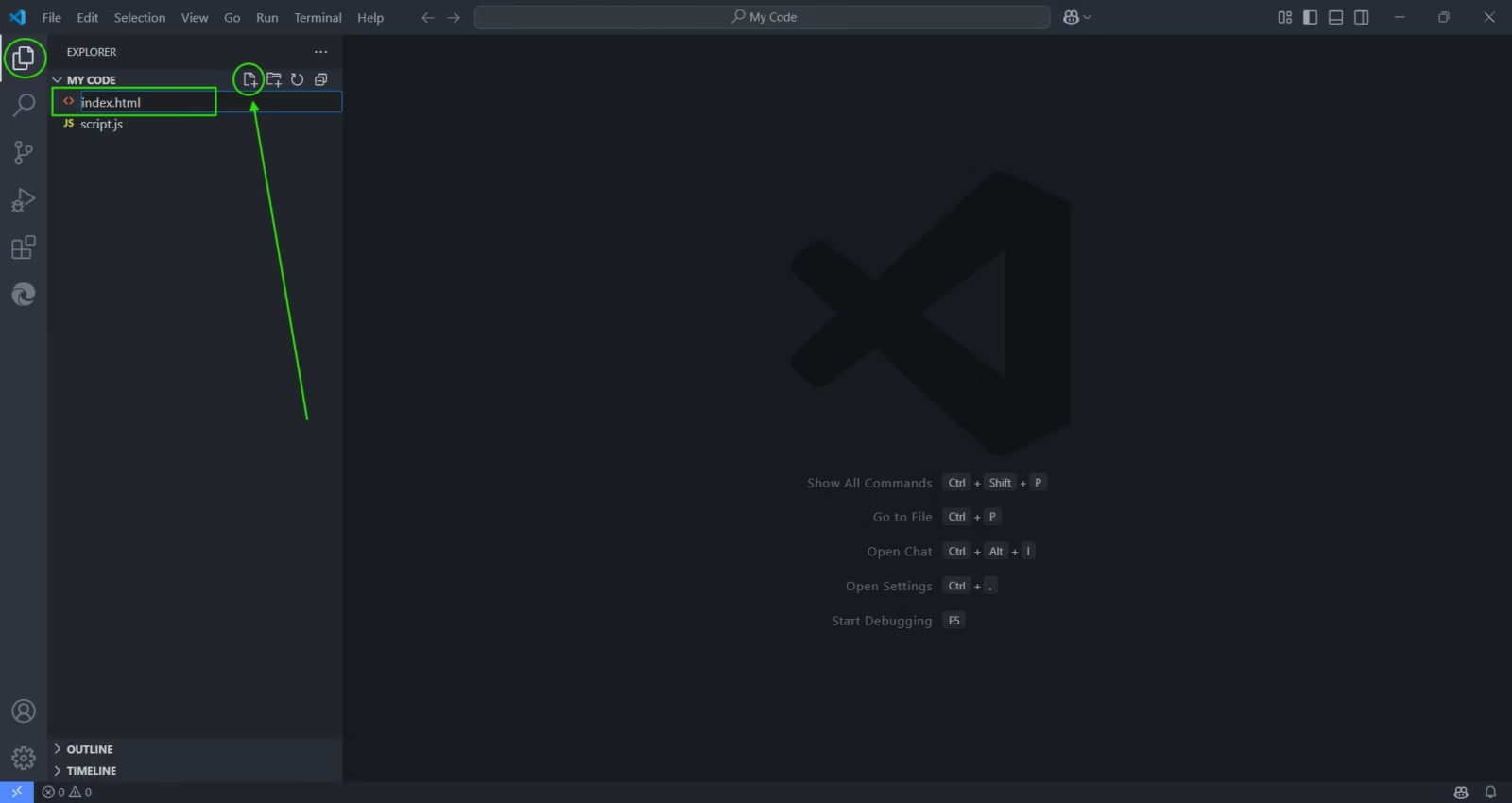
Now create an HTML Boilerplate. HTML Boilerplate is a template of HTML code which is used as a frame for the whole HTML file.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Hello World</title>
</head>
<body>
<h1>Hello World</h1>
<script src="script.js"></script>
</body>
</html>
As you can see in the screenshot below, there are 2 arrows, 1st one is for HTML code for Hello World and 2nd one is for connecting JavaScript files. To connect the JavaScript file we have added <script src="script.js"></script>
in <body>
section then we have used src="your-javascript-file-name"
attribute inside <script>
tag.
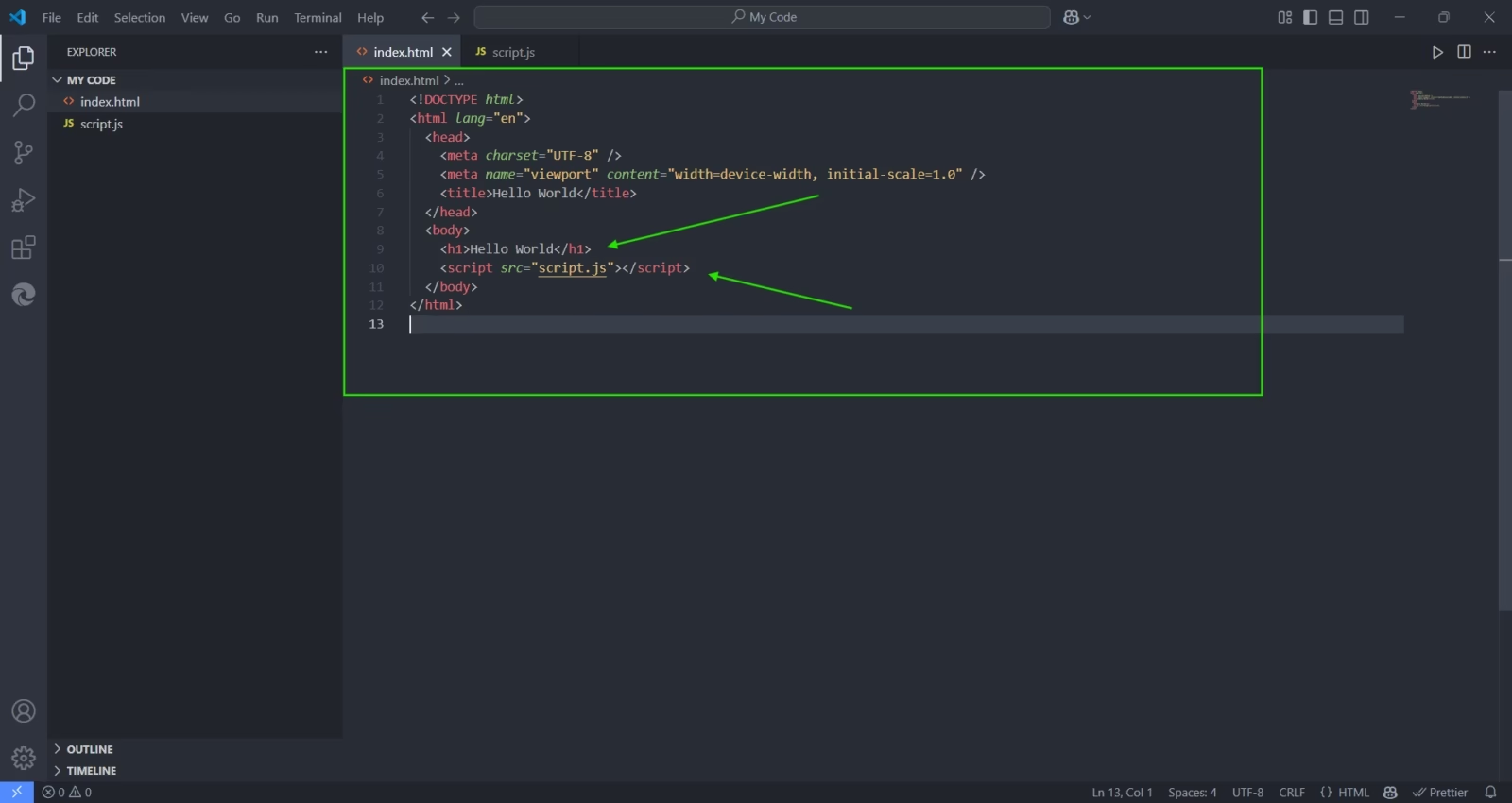
Now go to the JavaScript file that we have created in the previous section and write your JavaScript Code as given below. There are 2 pieces of JavaScript code which will show Hello World in 2 separate places.
console.log("Hello, World!"); // Hello World! will be printed to the console
alert("Hello, World!"); // Hello World! will be displayed in an alert box
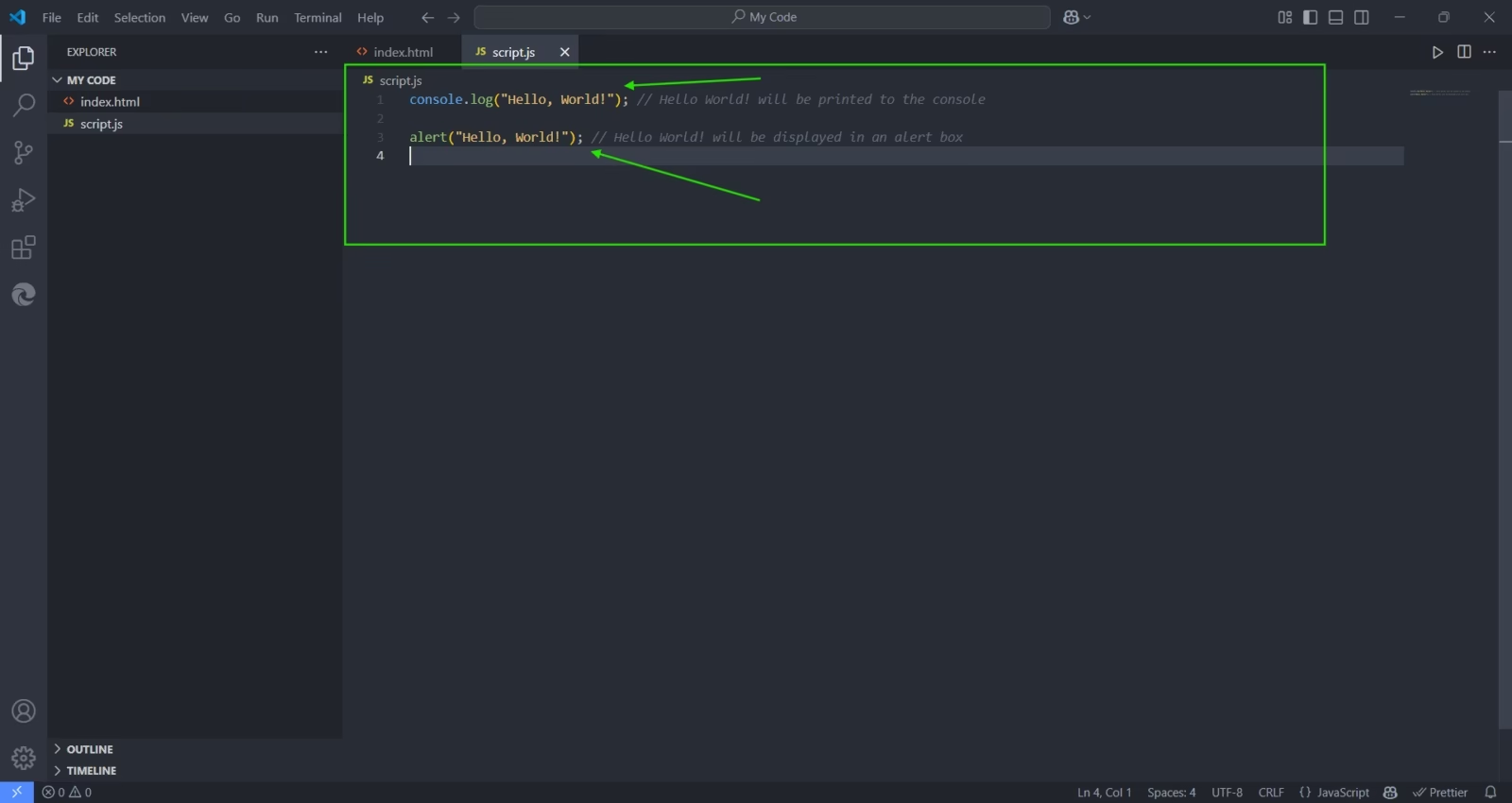
In Explorer, right-click on the HTML file and click on Copy Path. This will copy the file location. You have to paste the file location into your Web Browser.
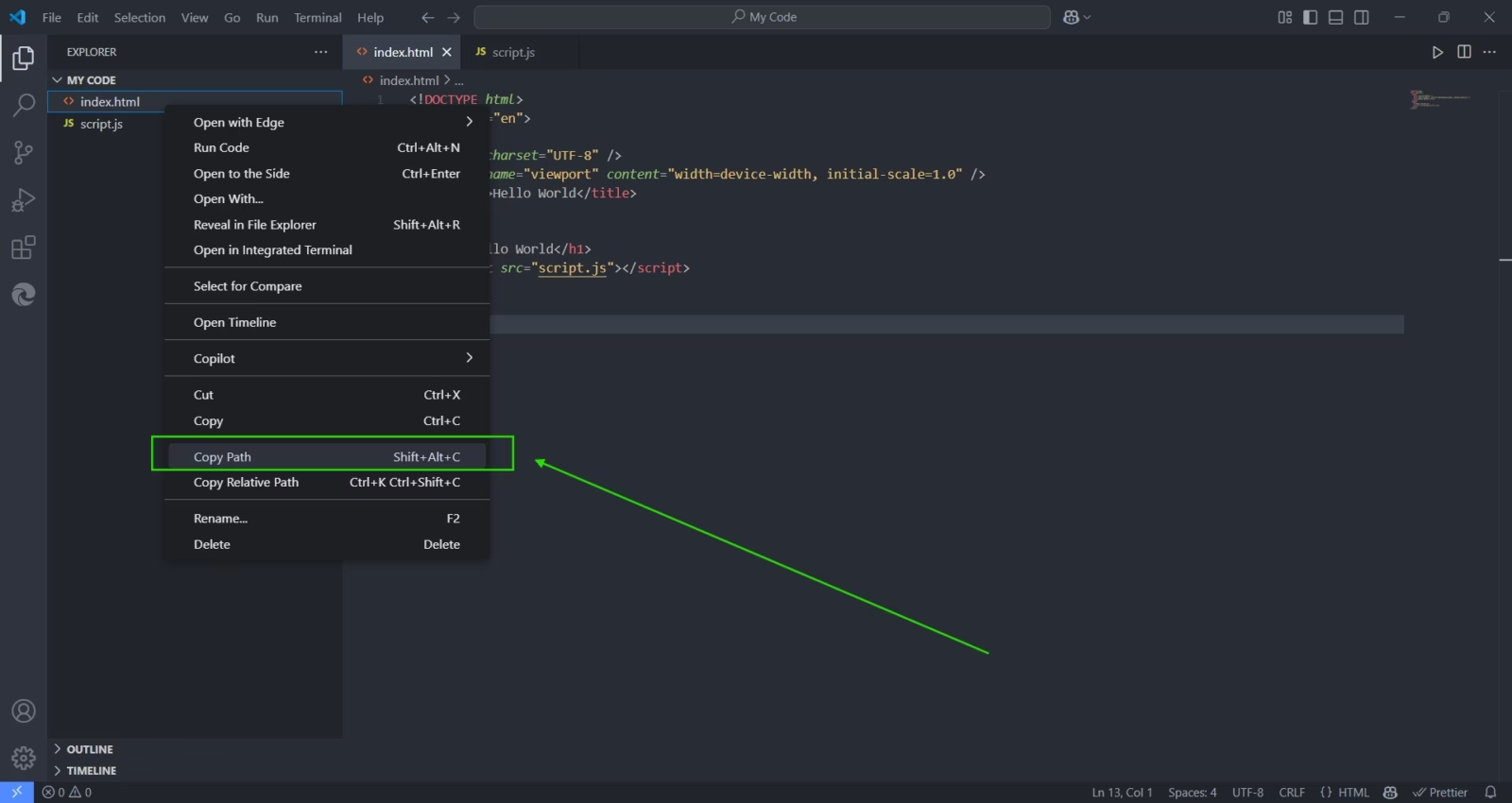
Open your Web Browser and paste the file location as shown in the screenshot below.
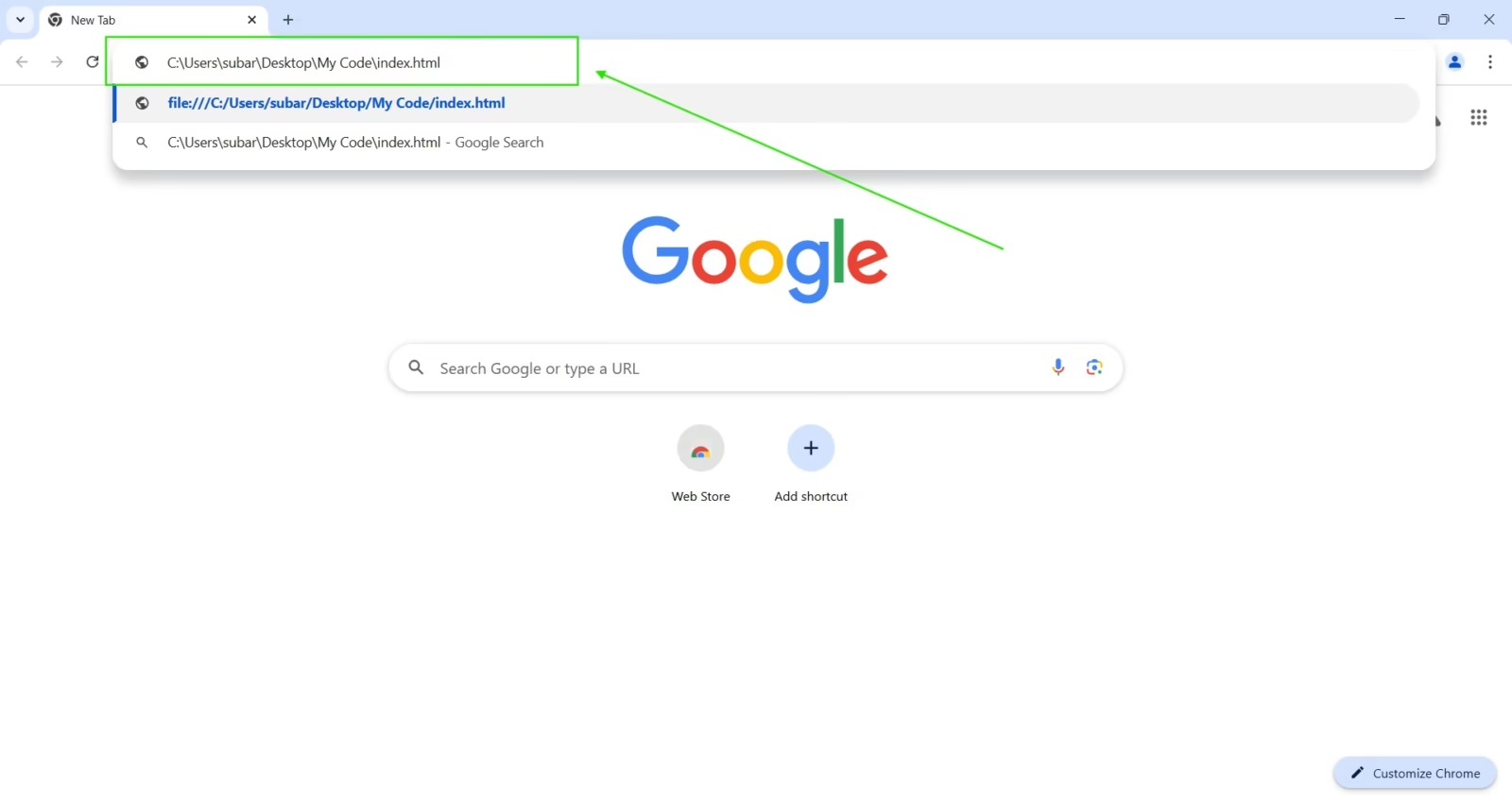
Now, the HTML file should open on your browser.
After opening the HTML file in your browser you’ll notice 2 different Hello World on the screen. Big and Bold Hello World is there because of the HTML <h1>
tag. The pop-up Hello World is there because of the alert()
JavaScript function. Another Hello World should be visible in the developer tool after you click OK on the pop-up window.
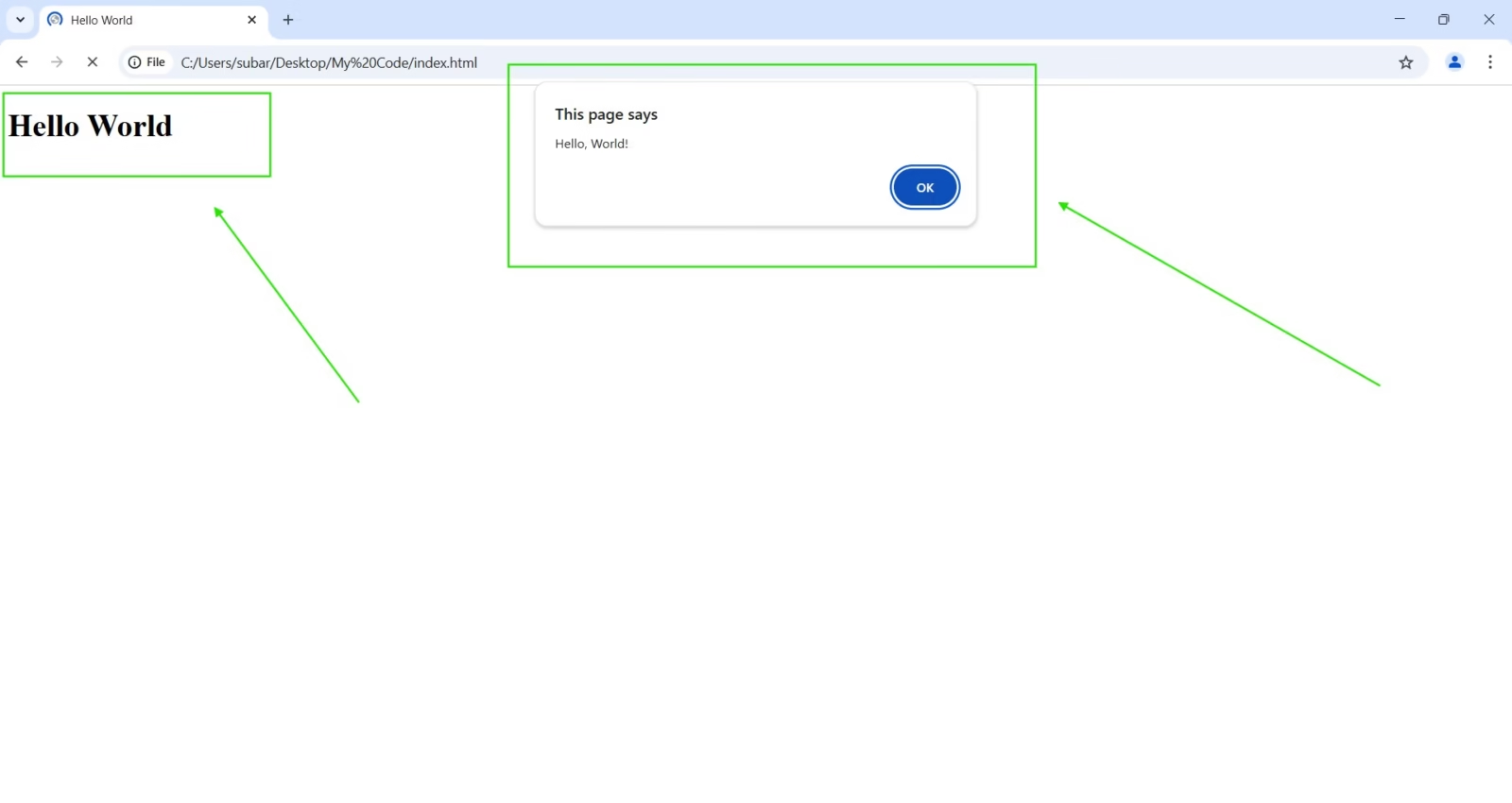
Click OK on the pop-up window then open the developer tool and click on Console (To open the developer tool, right-click on the screen and go to Inspect).
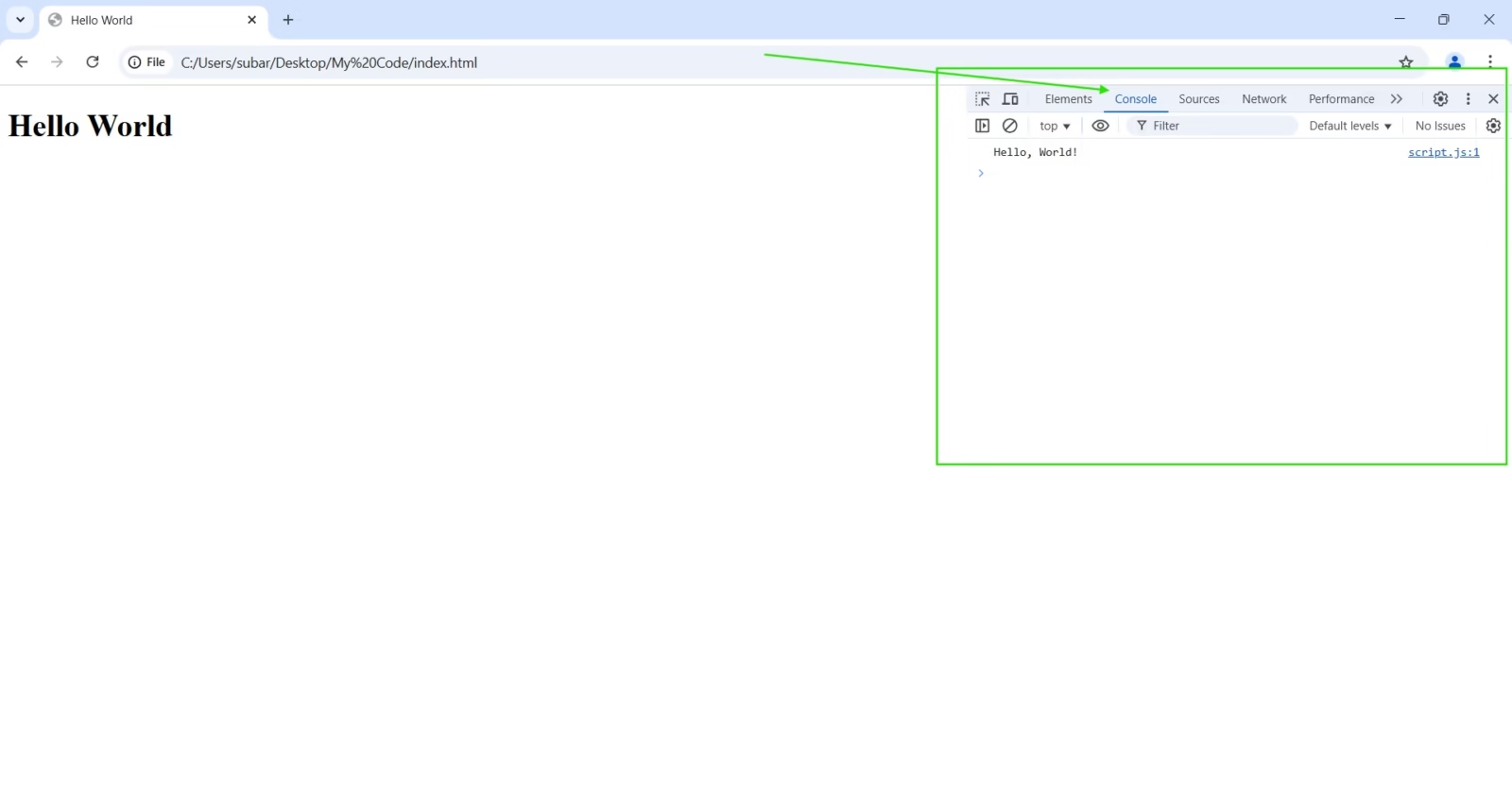
You have finished writing Hello World code in 3 different ways. Great work!
Best practices for beginners
Coding is not hard, but it is a lengthy work. You need to keep practising by writing the same code at least twice or thrice. Otherwise, you’ll surely forget what you learn.
Here we have shown you three ways to write the Hello World Program. Instead of Hello World, try writing a different message or note so that you remember how you wrote the code.
Conclusion
Creating Hello World program is crucial for beginners. It gives a general idea about how a piece of code works. Here we have mentioned 3 methods to write the Hello World program.
- By writing Hello World in the Developer console of the Web Browser.
- By writing Hello World in Code Editor and showing output in the Output tab.
- By linking the JavaScript file to the HTML document and opening the HTML file in Web Browser.
The first and the second methods are fairly easy as compared to the last one. First and Second methods have only 1 line of JavaScript code.
In the last method, we used the HTML Boilerplate as a frame to connect the JavaScript file using the <script> tag. Whenever you write HTML code you should always use a Boilerplate. You can use the Boilerplate we have shown here.
Since JavaScript always work with HTML in the front-end, you should always link JavaScript to the HTML file as we did in the last method. Using different files for JavaScript code and HTML code is recommended in most cases. This is known as external JavaScript. When you start JavaScript development, most of the time, you’ll use External JavaScript. This method will be useful for you for years to come.
We hope this post was helpful to you. Happy Coding!
Get up to 85% off
Build your project using Managed VPS & Dedicated hosting and decrease your workload.
You'll get seamless migration, exceptional savings, 24/7 technical support, HIPAA compliant, PCI compliant, Multi-Level DDoS protection, 30-day money-back guarantee and more with Liquid Web.
Affiliate Disclosure: Affiliate links are available here. If you click through the affiliate links and buy something, we may get a small commission, and you'll not be charged extra.