Compare Inline JavaScript vs External JavaScript to build your first project
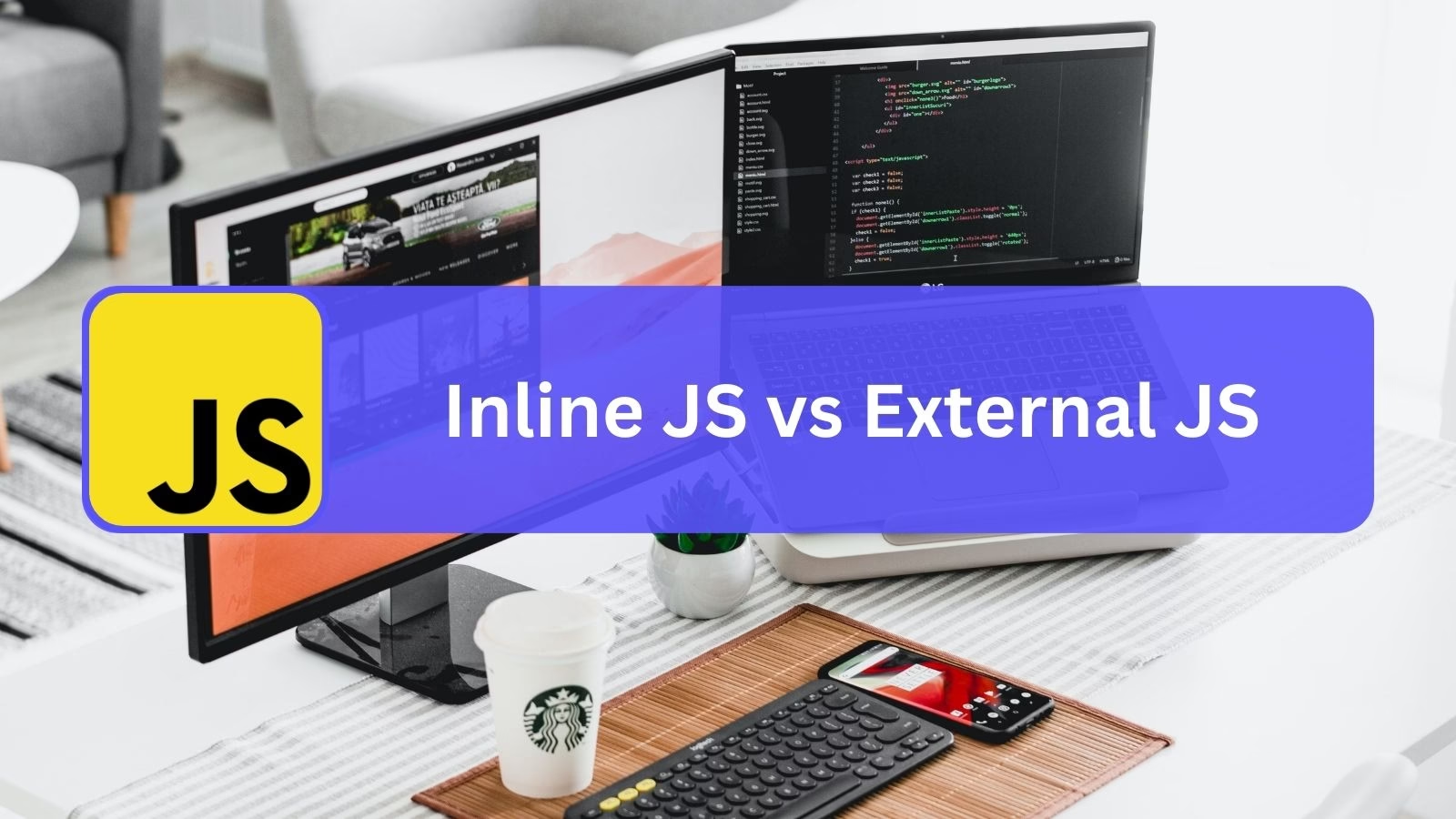
This post was generated with AI and then edited by us.
Here we’ll compare Inline JavaScript vs External JavaScript to understand their key differences, pros, and cons, helping you choose the right approach for your web development projects.
When you start learning with JavaScript, you’ll quickly encounter two ways of including your JavaScript code in an HTML document: using inline JavaScript or linking to external JavaScript files. Each approach has its strengths and is suitable for different situations. In this post, we’ll dive into both options, comparing them, and explore when to use one over the other.
What is Inline JavaScript?
Inline JavaScript refers to writing JavaScript code directly within an HTML document. You can place this code inside <script>
tags, typically at the bottom of the HTML document just before the closing </body>
tag. This method allows you to embed JavaScript directly within the HTML structure.
How to Add Inline JavaScript?
To add inline JavaScript, you use the <script>
tag in the HTML document. Everything inside the <script>
tag is JavaScript code.
Here’s a simple example of using inline JavaScript.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Inline JavaScript Example</title>
</head>
<body>
<h1>Welcome to Inline JavaScript</h1>
<!-- Inline JavaScript -->
<script>
console.log("This is inline JavaScript!");
</script>
</body>
</html>
What’s Happening in the Example?
- Inside the
<script>
tag, we wroteconsole.log("This is inline JavaScript!");
. This tells the browser to print a message in the developer console (where developers can check what’s happening on the website). - To open the developer console, right-click anywhere on the webpage and select “Inspect,” then go to the “Console” tab. There you’ll see “This is inline JavaScript!” printed.
Inline JavaScript: Pros and Cons
Let’s take a look at the advantages and disadvantages of using inline JavaScript.
Inline JavaScript Pros
- Simplicity: Inline JavaScript is quick and easy for small scripts or one-off functionality. It doesn’t require creating an additional file.
- Faster initial setup: If you’re just experimenting or working on a small project, adding code inline saves time.
Inline JavaScript Cons
- Limited Reusability: Inline JavaScript is usually specific to the current page. If you need to reuse the same script on other pages, you’d have to copy the code to each page, which can be cumbersome.
- Cluttered HTML: Having JavaScript mixed in with HTML can make your code harder to read and maintain, especially in larger projects.
- Performance Issues: While it’s not a huge concern for small scripts, embedding JavaScript inline can potentially slow down the loading time of your page because the browser needs to load and parse the JavaScript each time the page is accessed.
When to Use Inline JavaScript
Inline JavaScript can be ideal in the following situations:
- Quick Prototyping: If you’re building something small and don’t plan to reuse the code, inline JavaScript is a fast and simple solution.
- Page-Specific Logic: If the JavaScript code is only needed for a specific page and won’t be reused elsewhere, you can place the code directly in that page’s HTML.
- Simple Functions: If the JavaScript is doing something straightforward, like form validation or a simple user interaction, inline JavaScript works well.
What is External JavaScript?
External JavaScript involves writing JavaScript code in a separate .js
file, then linking to that file in your HTML document. This approach is beneficial for large projects where you have multiple pages or when you want to keep your JavaScript separate from the HTML for better organization.
How to Link an External JavaScript File to an HTML Document?
Step 1: Create Your External JavaScript File
To start, create a new file with a .js
extension. This will be your external JavaScript file. For example, you could name it script.js
. Write your JavaScript code inside this file.
Here’s an example of a simple JavaScript code inside script.js
:
// script.js
console.log("Hello, World!");
This script will log “Hello, World!” in the browser’s console.
Step 2: Link the JavaScript File in Your HTML Document
To link the external JavaScript file to your HTML document, you’ll need to use the <script>
tag in the HTML file.
Here’s the syntax for linking the external JavaScript file:
<script src="path-to-your-file.js"></script>
Example: Basic HTML Document with External JavaScript
Create an HTML file (e.g., index.html
) and link the external JavaScript file like so:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>External JavaScript Example</title>
</head>
<body>
<h1>External JavaScript Demo</h1>
<p>Check your browser console for the output.</p>
<!-- Link to external JavaScript file -->
<script src="script.js"></script>
</body>
</html>
In this example, the JavaScript file script.js
is linked using the <script>
tag. The src
attribute contains the path to the JavaScript file.
External JavaScript: Pros and Cons
External JavaScript offers several benefits for larger projects or when your codebase grows.
External JavaScript Pros
- Better Organization: Separating JavaScript from HTML improves readability and organization. It allows you to keep your HTML focused on structure and your JavaScript focused on functionality.
- Reusability: By using external JavaScript files, you can link to the same script on multiple pages. This is especially helpful if you want to apply the same functionality across your site.
- Cacheable: Browsers can cache external JavaScript files. Once the file is downloaded, it doesn’t need to be fetched again when a user navigates to other pages on your site, improving performance.
- Easier Debugging: With external files, you can easily use developer tools in the browser to debug your JavaScript, since the code is separated from the HTML content.
External JavaScript Cons
- Initial Setup Time: For small projects, setting up external JavaScript files might seem like overkill. It requires creating a separate file, writing the script there, and linking to it in your HTML.
- Dependency on File Location: If you move or rename your JavaScript file without updating the
src
path in your HTML, the script may fail to load.
When to Use External JavaScript
External JavaScript is better suited for the following scenarios:
- Larger Projects: When working on bigger websites or applications, keeping JavaScript in external files helps keep everything organized and modular.
- Code Reusability: If you have functionality that you want to reuse across multiple pages or even different projects, using external files makes the process more efficient.
- Performance: If you have a large script, it’s more efficient to cache it in the browser and load it only once, rather than having it embedded in every HTML file.
- Team Collaboration: In team projects, external JavaScript files make it easier to separate responsibilities. Developers can work on the JavaScript file while others work on the HTML and CSS.
Inline JavaScript vs External JavaScript
Feature | Inline JavaScript | External JavaScript |
---|---|---|
Code Location | Inside <script> tags in the HTML file | In a separate .js file |
Ease of Use | Easier for small projects or quick tests | Better for larger or reusable projects |
Reusability | Limited to the current page | Can be reused across multiple pages |
Organization | Mixes JavaScript with HTML | Keeps JavaScript separate from HTML |
Performance | Loads with every page request | Can be cached by the browser |
Team Collaboration | Harder to collaborate on mixed code | Easier to work on separate files |
Debugging | Debugging inline code is less structured | Easier with separate files and tools |
Setup Time | Quick initial setup | Requires creating and linking files |
Best Practices for JavaScript in HTML
Regardless of whether you use inline or external JavaScript, there are some best practices to follow:
Place Script Tags at the Bottom of the Page
When using inline JavaScript or external files, place the <script>
tag just before the closing </body>
tag to ensure the HTML content is loaded before the JavaScript runs. This avoids issues where your script tries to manipulate elements that haven’t been rendered yet.
<body>
<!-- Your content here -->
<script src="script.js"></script>
</body>
Use External Files for Larger Codebases
As your project grows, use external files for your JavaScript. It makes your code more maintainable and easier to manage.
Minify Your JavaScript
To improve performance, especially on production sites, minify your JavaScript files. This reduces the file size and speeds up loading times.
Conclusion
Both inline and external JavaScript have their place in web development, and the choice depends on the scale and complexity of your project. Inline JavaScript is useful for small, one-off scripts or quick experiments, while external JavaScript excels in larger projects, where code organization, reusability, and performance are crucial.
By understanding the strengths and limitations of each approach, you can choose the best one for your project and create clean, maintainable, and efficient web applications.
Happy coding!
Get up to 85% off
Build your project using Managed VPS & Dedicated hosting and decrease your workload.
You'll get seamless migration, exceptional savings, 24/7 technical support, HIPAA compliant, PCI compliant, Multi-Level DDoS protection, 30-day money-back guarantee and more with Liquid Web.
Affiliate Disclosure: Affiliate links are available here. If you click through the affiliate links and buy something, we may get a small commission, and you'll not be charged extra.