Guide to JavaScript Arithmetic using BODMAS/PEMDAS
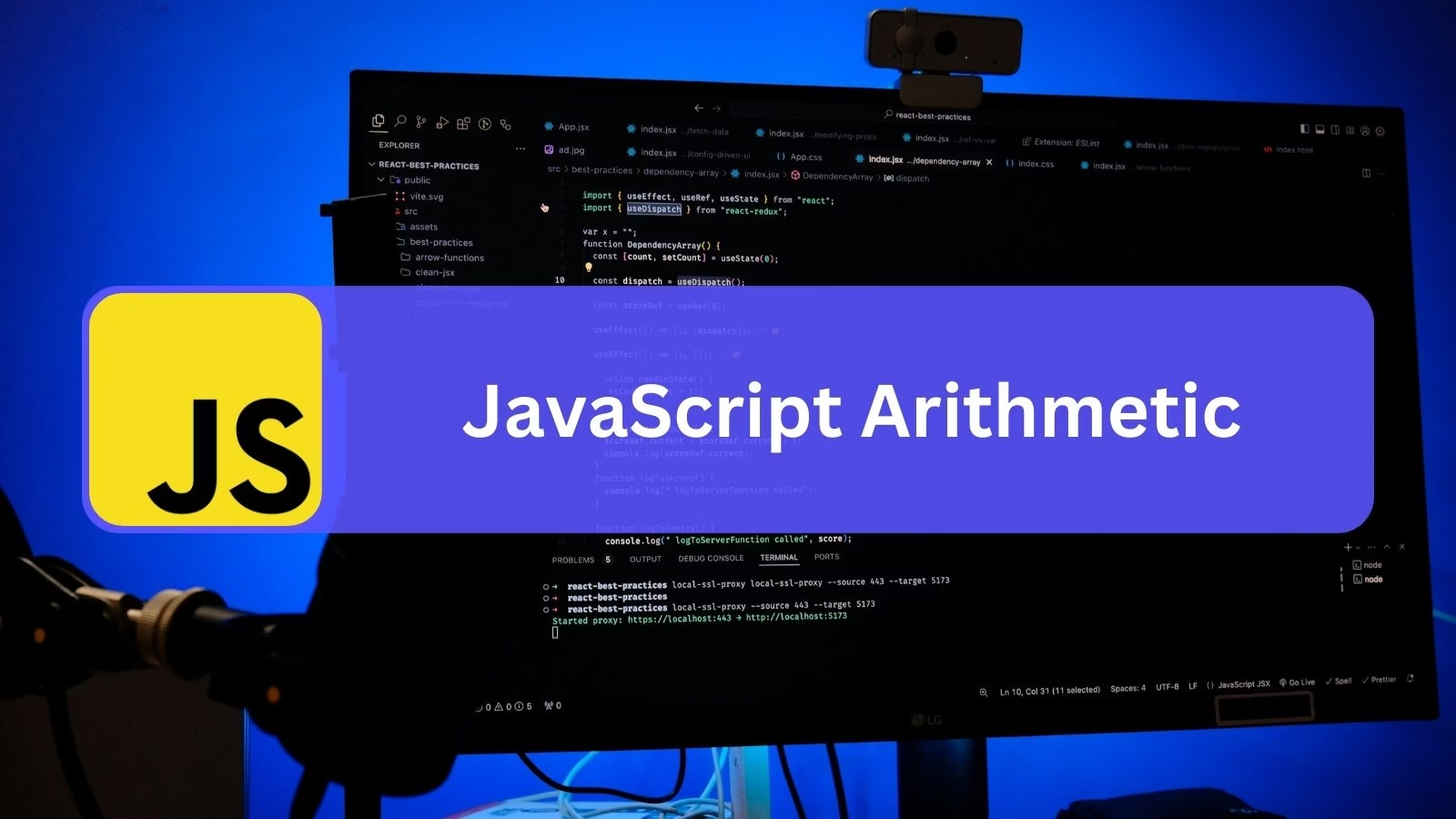
This post was generated with AI and then edited by us.
Welcome to the world of JavaScript! One of the essential building blocks of programming is understanding arithmetic operations. In this comprehensive guide, we’ll dive deep into JavaScript arithmetic, exploring each operation with detailed explanations and code examples. This guide is tailored for beginners, so we’ll keep the code simple and easy to follow. Let’s get started!
What is Arithmetic in JavaScript?
Arithmetic in JavaScript refers to performing mathematical operations such as addition, subtraction, multiplication, division, and more. These operations allow you to manipulate numbers and perform calculations in your code.
Basic Arithmetic Operators
JavaScript supports the following basic arithmetic operators:
- Addition (
+
) - Subtraction (
-
) - Multiplication (
*
) - Division (
/
) - Modulus (
%
) – Returns the remainder of a division - Exponentiation (
**
) – Raises the base to the power of the exponent - Increment (
++
): Increases a number by 1 - Decrement (
--
): Decreases a number by 1
Below is a table summarizing these operations:
Operation | Symbol | Description | Example | Result |
---|---|---|---|---|
Addition | + | Adds two numbers | 5 + 3 | 8 |
Subtraction | - | Subtracts one number from another | 5 - 3 | 2 |
Multiplication | * | Multiplies two numbers | 5 * 3 | 15 |
Division | / | Divides one number by another | 6 / 3 | 2 |
Modulus | % | Returns the remainder of a division | 7 % 3 | 1 |
Exponentiation | ** | Raises the base to the power of exponent | 2 ** 3 | 8 |
Increment | ++ | Increases a number by 1 | let a = 5; a++; | 6 |
Decrement | -- | Decreases a number by 1 | let b = 5; b--; | 4 |
Detailed Examples and Explanations of JavaScript Arithmetic Operators
1. Addition (+
)
Addition is used to sum two or more numbers.
Example:
let a = 5;
let b = 3;
let sum = a + b;
console.log("Sum: " + sum); // Output: Sum: 8
Explanation:
In this example, we declare two variables a
and b
, and use the +
operator to add them together. The result is stored in the sum
variable and printed to the console.
2. Subtraction (-
)
Subtraction is used to find the difference between two numbers.
Example:
let a = 5;
let b = 3;
let difference = a - b;
console.log("Difference: " + difference); // Output: Difference: 2
Explanation:
Here, we use the -
operator to subtract b
from a
. The result is stored in the difference
variable and printed to the console.
3. Multiplication (*
)
Multiplication is used to find the product of two numbers.
Example:
let a = 5;
let b = 3;
let product = a * b;
console.log("Product: " + product); // Output: Product: 15
Explanation:
We use the *
operator to multiply a
and b
. The result is stored in the product
variable and printed to the console.
4. Division (/
)
Division is used to divide one number by another.
Example:
let a = 6;
let b = 3;
let quotient = a / b;
console.log("Quotient: " + quotient); // Output: Quotient: 2
Explanation:
In this example, we use the /
operator to divide a
by b
. The result is stored in the quotient
variable and printed to the console.
5. Modulus (%
)
The modulus operator returns the remainder of a division.
Example:
let a = 7;
let b = 3;
let remainder = a % b;
console.log("Remainder: " + remainder); // Output: Remainder: 1
Explanation:
Here, we use the %
operator to find the remainder when a
is divided by b
. The result is stored in the remainder
variable and printed to the console.
6. Exponentiation (**
)
Exponentiation raises the base to the power of the exponent.
Example:
let a = 2;
let b = 3;
let power = a ** b;
console.log("Power: " + power); // Output: Power: 8
Explanation:
In this example, we use the **
operator to raise a
to the power of b
. The result is stored in the power
variable and printed to the console.
7. Increment and Decrement Operators
JavaScript also provides increment (++
) and decrement (--
) operators for adding or subtracting 1 from a variable.
Example:
let count = 0;
count++; // Increment count by 1
console.log("Incremented Count: " + count); // Output: Incremented Count: 1
count--; // Decrement count by 1
console.log("Decremented Count: " + count); // Output: Decremented Count: 0
Explanation:
The ++
operator increases the variable count
by 1, while the --
operator decreases it by 1.
Combining Arithmetic Operations
You can combine multiple arithmetic operations in a single expression. Use parentheses to control the order of operations, following the standard mathematical rules (PEMDAS/BODMAS).
Example:
let result = (5 + 3) * (10 / 2) - 4;
console.log("Result: " + result); // Output: Result: 28
Explanation:
In this example, parentheses ensure that addition and division are performed first, followed by multiplication and subtraction. This results in a calculated result
of 28.
Certainly! Let’s dive into the concepts of BODMAS (also known as PEMDAS) and apply them to JavaScript arithmetic through a real-life code challenge.
Understanding BODMAS/PEMDAS
BODMAS and PEMDAS are acronyms that represent the order of operations in mathematics:
- BODMAS stands for Brackets, Orders (i.e., powers and roots, etc.), Division and Multiplication, Addition and Subtraction.
- PEMDAS stands for Parentheses, Exponents, Multiplication and Division, Addition and Subtraction.
The order of operations dictates the sequence in which calculations should be performed to ensure accurate results. Here’s how it works in JavaScript:
- Parentheses/Brackets (
()
or{}
): Operations inside parentheses are performed first. - Exponents/Orders (
**
): Exponentiation comes next. - Multiplication and Division (
*
,/
): These operations are performed from left to right. - Addition and Subtraction (
+
,-
): These operations are performed last, from left to right.
Real Life example of JavaScript Arithmetic using BODMAS/PEMDAS
Imagine you want to create a simple program to calculate your monthly budget. You have expenses for rent, groceries, utilities, and entertainment. You also have income from your job and a side gig. Let’s use JavaScript to calculate your net savings for the month, applying the BODMAS/PEMDAS rules.
Here’s how we can approach this problem:
- Calculate the total monthly expenses.
- Calculate the total monthly income.
- Subtract the total expenses from the total income to find the net savings.
JavaScript Code Example
// Monthly expenses
let rent = 1500;
let groceries = 300;
let utilities = 200;
let entertainment = 100;
// Total monthly expenses
let totalExpenses = rent + groceries + utilities + entertainment;
console.log("Total Expenses: $" + totalExpenses); // Output: Total Expenses: $2100
// Monthly income
let jobIncome = 3500;
let sideGigIncome = 500;
// Total monthly income
let totalIncome = jobIncome + sideGigIncome;
console.log("Total Income: $" + totalIncome); // Output: Total Income: $4000
// Net savings calculation
let netSavings = totalIncome - totalExpenses;
console.log("Net Savings: $" + netSavings); // Output: Net Savings: $1900
// Applying BODMAS/PEMDAS rules explicitly
let complexCalculation = (jobIncome + sideGigIncome) - (rent + groceries + utilities + entertainment);
console.log("Net Savings (using BODMAS): $" + complexCalculation); // Output: Net Savings (using BODMAS): $1900
// Example with mixed operations
let mixedOperations = (totalIncome / 2) ** 2 - (rent + groceries) * (utilities - entertainment);
console.log("Result of mixed operations: " + mixedOperations);
Explanation of the Code
- Calculating Total Expenses: We sum up the monthly expenses for rent, groceries, utilities, and entertainment using the
+
operator. - Calculating Total Income: We sum up the monthly income from the job and the side gig using the
+
operator. - Calculating Net Savings: We subtract the total expenses from the total income to find the net savings for the month.
- Applying BODMAS/PEMDAS: We explicitly use parentheses to ensure the correct order of operations in the
complexCalculation
andmixedOperations
variables.
Using Arithmetic Operators in Functions
Using arithmetic operators within functions is a fundamental aspect of JavaScript programming. Functions allow you to encapsulate reusable code, and arithmetic operations within these functions enable you to perform calculations dynamically.
Let’s explore how to use arithmetic operators in functions with a few examples:
Example 1: Basic Arithmetic Operations in a Function
In this example, we’ll create a function that performs basic arithmetic operations (addition, subtraction, multiplication, and division) on two numbers.
// Function to perform basic arithmetic operations
function basicArithmetic(a, b) {
let addition = a + b;
let subtraction = a - b;
let multiplication = a * b;
let division = a / b;
console.log("Addition: " + addition);
console.log("Subtraction: " + subtraction);
console.log("Multiplication: " + multiplication);
console.log("Division: " + division);
}
// Calling the function with example values
basicArithmetic(10, 5);
Explanation:
- The
basicArithmetic
function takes two parametersa
andb
. - Within the function, we perform addition, subtraction, multiplication, and division operations.
- The results are stored in variables (
addition
,subtraction
,multiplication
, anddivision
) and printed to the console.
Example 2: Calculating the Area of a Rectangle
In this example, we’ll create a function to calculate the area of a rectangle using the multiplication operator.
// Function to calculate the area of a rectangle
function calculateArea(length, width) {
return length * width;
}
// Calling the function with example values
let length = 10;
let width = 5;
let area = calculateArea(length, width);
console.log("Area of the Rectangle: " + area); // Output: Area of the Rectangle: 50
Explanation:
- The
calculateArea
function takes two parameterslength
andwidth
. - The function multiplies the length and width to calculate the area and returns the result.
- We call the function with example values and store the result in the
area
variable, which is then printed to the console.
Example 3: Using Exponentiation in Functions
In this example, we’ll create a function to calculate the power of a number using the exponentiation operator.
// Function to calculate the power of a number
function calculatePower(base, exponent) {
return base ** exponent;
}
// Calling the function with example values
let base = 2;
let exponent = 3;
let result = calculatePower(base, exponent);
console.log("Result: " + result); // Output: Result: 8
Explanation:
- The
calculatePower
function takes two parametersbase
andexponent
. - The function raises the base to the power of the exponent using the
**
operator and returns the result. - We call the function with example values and store the result in the
result
variable, which is then printed to the console.
Example 4: Calculating Average of an Array
In this example, we’ll create a function to calculate the average of an array of numbers using addition and division operators.
// Function to calculate the average of an array of numbers
function calculateAverage(numbers) {
let total = 0;
for (let i = 0; i < numbers.length; i++) {
total += numbers[i];
}
return total / numbers.length;
}
// Calling the function with an example array
let numbersArray = [10, 20, 30, 40, 50];
let average = calculateAverage(numbersArray);
console.log("Average: " + average); // Output: Average: 30
Explanation:
- The
calculateAverage
function takes an arraynumbers
as a parameter. - The function initializes a
total
variable to 0 and uses a loop to add each element of the array to thetotal
. - The function returns the average by dividing the
total
by the number of elements in the array (numbers.length
). - We call the function with an example array and store the result in the
average
variable, which is then printed to the console.
Example 5: Increment and Decrement in Functions
In this example, we’ll create functions to increment and decrement a number.
// Function to increment a number
function incrementNumber(num) {
return num + 1;
}
// Function to decrement a number
function decrementNumber(num) {
return num - 1;
}
// Calling the functions with example values
let number = 10;
let incrementedNumber = incrementNumber(number);
let decrementedNumber = decrementNumber(number);
console.log("Incremented Number: " + incrementedNumber); // Output: Incremented Number: 11
console.log("Decremented Number: " + decrementedNumber); // Output: Decremented Number: 9
Explanation:
- The
incrementNumber
function takes a parameternum
and returns the value ofnum
incremented by 1. - The
decrementNumber
function takes a parameternum
and returns the value ofnum
decremented by 1. - We call the functions with an example value and store the results in
incrementedNumber
anddecrementedNumber
variables, which are then printed to the console.
Best Practices for JavaScript Arithmetic
To make sure your JavaScript arithmetic code is efficient, readable, and maintainable, here are some best practices to follow:
1. Use Descriptive Variable Names
Use clear and meaningful variable names that describe the purpose of the variable. This makes your code easier to understand and maintain.
// Poor practice
let x = 10;
let y = 5;
let z = x + y;
// Good practice
let length = 10;
let width = 5;
let area = length + width;
2. Use Comments Wisely
Add comments to explain complex calculations or the purpose of the code. Avoid over-commenting or stating the obvious.
// Calculate the area of a rectangle
let length = 10;
let width = 5;
let area = length * width; // Area is length multiplied by width
3. Be Mindful of Number Types
JavaScript has only one type of number (double-precision floating-point format). Be careful when working with floating-point numbers to avoid precision issues.
let result = 0.1 + 0.2;
console.log(result); // Output: 0.30000000000000004
To handle precision issues, consider using libraries like Big.js
or Decimal.js
.
4. Use Parentheses for Clarity
Use parentheses to clearly define the order of operations, even if it follows the default precedence rules. This improves readability and reduces the chances of errors.
// Without parentheses
let result = 5 + 3 * 2; // Result: 11 (Multiplication first, then addition)
// With parentheses
let result = (5 + 3) * 2; // Result: 16 (Addition first, then multiplication)
5. Avoid Magic Numbers
Magic numbers are hard-coded values with no explanation. Use constants or variables with descriptive names instead.
// Poor practice
let totalCost = 100 + 20 * 0.08;
// Good practice
const TAX_RATE = 0.08;
let itemCost = 100;
let shippingCost = 20;
let totalCost = itemCost + shippingCost * TAX_RATE;
6. Handle Division by Zero
Always check for division by zero to prevent runtime errors or unexpected behavior.
let divisor = 0;
if (divisor !== 0) {
let result = 10 / divisor;
console.log(result);
} else {
console.log("Cannot divide by zero");
}
7. Use the Increment and Decrement Operators Appropriately
Be aware of the difference between pre-increment (++var
) and post-increment (var++
), as well as pre-decrement (--var
) and post-decrement (var--
).
let count = 0;
console.log(count++); // Output: 0 (Post-increment)
console.log(count); // Output: 1
count = 0;
console.log(++count); // Output: 1 (Pre-increment)
console.log(count); // Output: 1
8. Leverage Built-in Math Functions
JavaScript’s Math
object provides various mathematical functions that can simplify your code and improve accuracy.
let value = -10;
console.log(Math.abs(value)); // Output: 10 (Absolute value)
let radians = Math.PI / 4;
console.log(Math.sin(radians)); // Output: 0.7071067811865475 (Sine of 45 degrees)
9. Minimize Global Variables
Avoid using global variables for arithmetic operations, as they can lead to code that is difficult to debug and maintain. Encapsulate calculations within functions or objects.
// Poor practice
let total = 0;
function addToTotal(value) {
total += value;
}
// Good practice
function calculateTotal(value1, value2) {
return value1 + value2;
}
let total = calculateTotal(100, 200);
10. Use Constants for Fixed Values
Use const
to declare constants for values that do not change. This ensures that these values are not accidentally modified.
const PI = 3.14159;
let radius = 5;
let circumference = 2 * PI * radius;
Conclusion
Understanding arithmetic operators is fundamental for working with JavaScript. These operations allow you to perform a wide range of mathematical calculations, from simple addition to complex equations. As you continue learning JavaScript, you’ll find that these basic arithmetic operations are essential building blocks for more advanced programming concepts.
Happy coding!
Get up to 85% off
Build your project using Managed VPS & Dedicated hosting and decrease your workload.
You'll get seamless migration, exceptional savings, 24/7 technical support, HIPAA compliant, PCI compliant, Multi-Level DDoS protection, 30-day money-back guarantee and more with Liquid Web.
Affiliate Disclosure: Affiliate links are available here. If you click through the affiliate links and buy something, we may get a small commission, and you'll not be charged extra.
Great Post