Decoding JavaScript Variables: Your Guide to the Building Blocks of Code
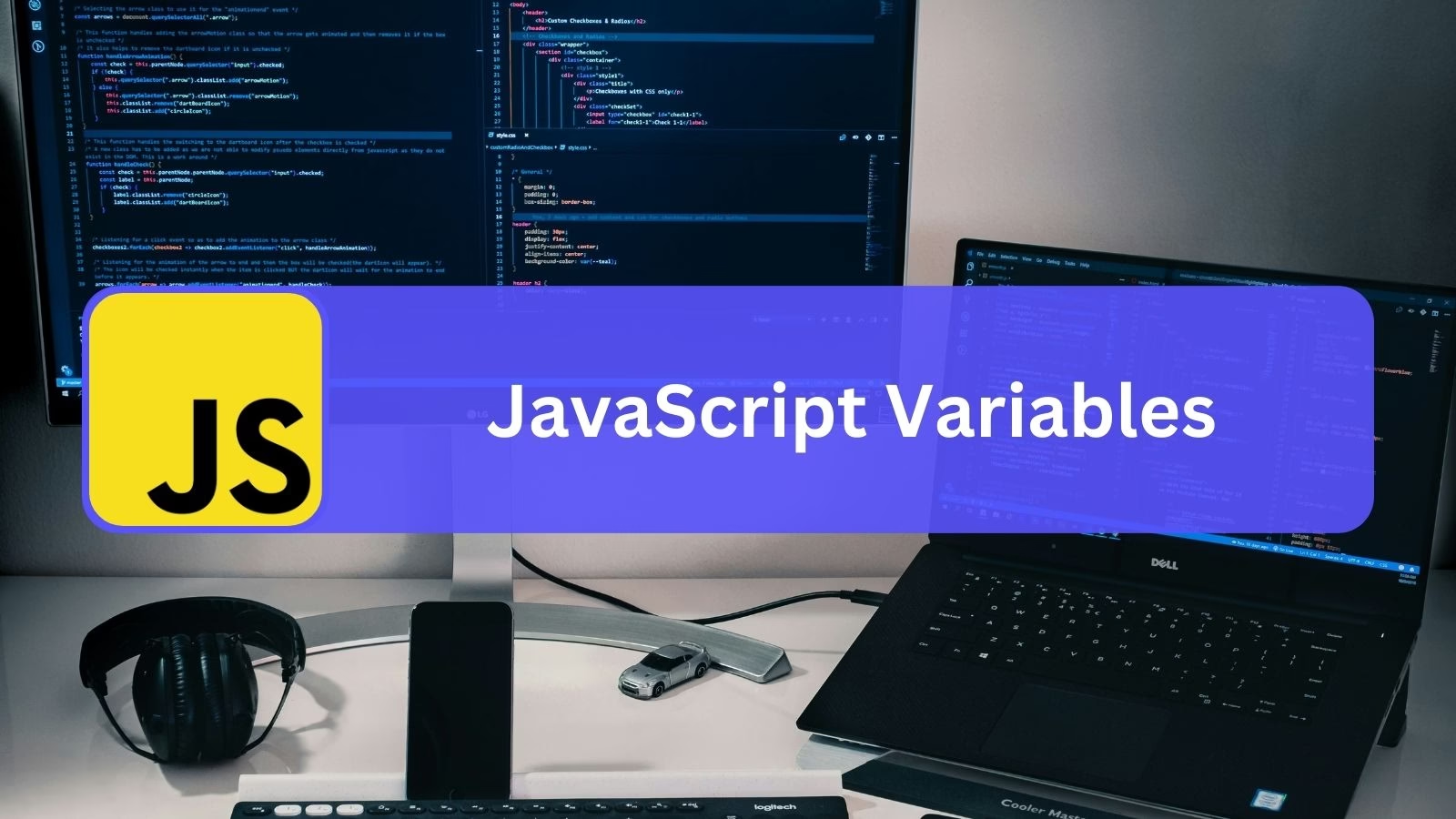
This post was generated with AI and then edited by us.
JavaScript, the dynamic scripting language that powers countless web experiences, relies heavily on variables. Think of them as labelled containers that hold data – information your program needs to work with. Understanding variables is absolutely fundamental to mastering JavaScript.
This post will break down everything you need to know, from declaration and initialization to scope and best practices.
What are JavaScript Variables?
At their core, variables are named storage locations in your computer’s memory. You use them to store different types of data, like numbers, text, or even more complex structures. The “variable” part means the value stored within these containers can change during the execution of your program.
Think of variables as labelled boxes that store information. This information, or “data,” can be of different types, such as numbers, text, or even complex structures like arrays and objects.
With variables, you can easily reuse and manipulate data in your program.
Declaring Variables
Before you can use a variable, you need to declare it. In modern JavaScript, we primarily use three keywords for this:
let
: Introduced in ES6 (ECMAScript 2015),let
is the preferred way to declare variables. It offers block scope, which we’ll discuss later.const
: Also from ES6,const
declares a constant. This means the value assigned to it cannot be re-assigned later (although the properties of an object assigned to aconst
can be modified).var
: The older way of declaring variables. While still functional,var
has some quirks related to scope, makinglet
andconst
generally better choices.
Here’s how you declare a variable:
let myName; // Declares a variable named 'myName' using let
const PI = 3.14159; // Declares a constant named 'PI' using const
var age; // Declares a variable named 'age' using var
Initializing Variables: Giving them value
Declaring a variable simply creates the container. To actually store data in it, you need to initialize it by assigning a value. You can do this at the time of declaration or later.
let myName = "John Doe"; // Declares and initializes 'myName' with the string "John Doe"
const PI = 3.14159; // Declares and initializes 'PI' with the number 3.14159
var age;
age = 30; // Initializes 'age' with the number 30 later
How to use const
& let
variables in JavaScript?
const
and let
are modern ways to declare variables in JavaScript, introduced in ES6 (ECMAScript 2015). They offer significant improvements over the older var
keyword, especially regarding scope and how values can be reassigned. Let’s break down how to use them:
const
(Constants)
Purpose: const
declares a constant. This means that once you assign a value to a const
variable, you cannot reassign it to a new value. This is important for values that should remain unchanged throughout your program’s execution.
Declaration and Initialization: You must initialize a const
variable when you declare it. You cannot declare it without assigning a value.
const PI = 3.14159; // Correct: Declaration and initialization together
// const E; // Incorrect: Missing initialization; this will cause an error.
Reassignment: Attempting to reassign a const
variable will result in a TypeError
.
const PI = 3.14159;
// PI = 3.14; // This will throw an error: "Assignment to constant variable."
Scope: const
has block scope. This means it’s only accessible within the block of code (e.g., a function, loop, or if
statement) where it’s defined.
function myFunction() {
const message = "Hello!";
console.log(message); // Accessible here
}
myFunction();
// console.log(message); // Not accessible here (outside the function)
Object Immutability (Important Nuance): While you can’t reassign a const
variable to a new value, if that value is an object (including arrays), you can modify the properties of that object.
const myObject = { name: "Alice", age: 30 };
myObject.age = 31; // This is allowed! We're modifying a property, not reassigning the object.
console.log(myObject); // Output: { name: "Alice", age: 31 }
// myObject = { name: "Bob", age: 25 }; // This is NOT allowed (reassignment)
const myArray = [1, 2, 3];
myArray.push(4); // This is allowed! Modifying the array.
console.log(myArray); // Output: [1, 2, 3, 4]
// myArray = [5, 6, 7]; // This is NOT allowed.
let
(Block-Scoped Variables)
Purpose: let
declares variables that you can reassign. It’s the more modern replacement for var
.
Declaration and Initialization: You can declare a let
variable without immediately initializing it. If you do this, its initial value will be undefined
.
let name; // Declares 'name' (value is undefined)
name = "Bob"; // Initializes 'name' later
let count = 0; // Declares and initializes 'count'
Reassignment: You can reassign let
variables as many times as you need.
let counter = 0;
counter = 1;
counter = counter + 5; // Now counter is 6
Scope: Like const
, let
has block scope. This is a major advantage over var
, which has function scope. Block scope helps prevent accidental variable overwrites and makes your code more predictable.
function myFunction() {
let x = 10;
if (true) {
let y = 20;
console.log(x, y); // x and y are accessible here
}
console.log(x); // x is accessible here, but y is NOT (block scope)
}
myFunction();
Key differences and when to use const
and let
Feature | const | let |
---|---|---|
Reassignment | Not allowed (except for object properties) | Allowed |
Initialization | Required at declaration | Optional at declaration |
Scope | Block scope | Block scope |
- Use
const
by default: If you know a variable’s value won’t change,const
is the best choice. It makes your code more readable and helps prevent accidental modifications. - Use
let
when you need to reassign: If you anticipate needing to change a variable’s value, uselet
. - Avoid
var
: Unless you have a specific reason to use it (e.g., compatibility with very old code), preferconst
andlet
for their better scope management.
Code demonstration of const
and let
.
const MAX_VALUE = 100; // Constant (shouldn't change)
let currentScore = 0; // Variable that will change
currentScore = 50; // Updating the score
if (currentScore > MAX_VALUE) {
console.log("New High Score!");
// MAX_VALUE = currentScore; // This would be an error!
}
let message = "Hello!";
message = "Goodbye!"; // Reassigning message is fine with let.
console.log(message); // Output: Goodbye!
By understanding const
and let
, you’ll write cleaner, more maintainable, and less error-prone JavaScript code. The block scope provided by these keywords is a crucial improvement over var
.
Data types in Variable: What Can Variables Hold?
JavaScript is a dynamically typed language, meaning you don’t explicitly specify the data type of a variable when you declare it. JavaScript infers the type based on the value you assign. Some common data types include:
- String: Textual data (e.g., “Hello, World!”)
- Number: Numeric data (e.g., 10, 3.14)
- Boolean: True or false values
- Null: Represents the intentional absence of a value
- Undefined: Represents a variable that has been declared but not yet assigned a value
- Object: Complex data structures that can hold multiple values
- Symbol: Unique and immutable values (often used as keys in objects)
- BigInt: Represents integers of arbitrary precision
To learn more about data types in JavaScript visit MDN web docs.
JavaScript variables with real-world code examples
The examples given below illustrate how variables are used in different scenarios. They store various types of data (strings, numbers, booleans) and are essential for performing calculations, making decisions, and managing data in your JavaScript programs. Remember to use descriptive names for your variables and choose the appropriate declaration keyword (let
or const
).
User profile
Imagine you’re building a simple user profile page. You’d use variables to store user information.
let userName = "Alice"; // String for the user's name
const userAge = 30; // Number for the user's age (we assume it won't change)
let isLoggedIn = true; // Boolean indicating if the user is logged in
let userBio = ""; // String for the user's bio (starts empty)
// Displaying the information:
console.log("Welcome, " + userName + "!");
console.log("Age: " + userAge);
if (isLoggedIn) {
console.log("You are currently logged in.");
} else {
console.log("Please log in to view more.");
}
// Later, the user might update their bio:
userBio = "I love coding and hiking!";
console.log("Updated Bio: " + userBio);
// Or, if they log out:
isLoggedIn = false;
console.log("User logged out. isLoggedIn is now: " + isLoggedIn);
Here, userName
, userAge
, isLoggedIn
, and userBio
are variables holding different pieces of user data. We use let
for information that might change (like userBio
and isLoggedIn
) and const
for information we expect to remain constant (like userAge
).
E-commerce shopping cart
Let’s simulate a simplified shopping cart.
let productName = "Laptop";
let productPrice = 1200;
let quantity = 2;
let totalPrice = productPrice * quantity; // Calculate the total price
console.log("You have " + quantity + " " + productName + "s in your cart.");
console.log("Total price: $" + totalPrice);
// Applying a discount:
let discountPercentage = 10;
let discountAmount = totalPrice * (discountPercentage / 100);
totalPrice = totalPrice - discountAmount; // Update the total price
console.log("Discount applied: $" + discountAmount);
console.log("Final price: $" + totalPrice);
// Adding another item:
let anotherProductName = "Mouse";
let anotherProductPrice = 25;
let anotherQuantity = 1;
// We could add this new item to the cart's data structure (e.g., an array), but for simplicity, let's calculate a grand total
let grandTotal = totalPrice + (anotherProductPrice * anotherQuantity);
console.log("Grand Total: $" + grandTotal);
In this example, variables store product information, quantities, and calculate prices. We use variables to update the cart’s total after applying a discount.
Simple game score
Let’s create a basic game score system.
let playerScore = 0;
let highScore = 100;
// Player scores some points:
let pointsEarned = 25;
playerScore = playerScore + pointsEarned; // Update the player's score
console.log("Player Score: " + playerScore);
// Check if the player beat the high score:
if (playerScore > highScore) {
highScore = playerScore; // Update the high score
console.log("New High Score! " + highScore);
}
// Game over, reset the player's score for the next round:
playerScore = 0;
console.log("Game Over. Player score reset to: " + playerScore);
Here, variables track the player’s score and the high score. We update the player’s score and check if they’ve achieved a new high score.
Form validation
Variables can be used to store form input and check if the input is valid.
let email = "test@example.com"; // Get the email input from the form
let isValidEmail = email.includes("@"); // A simplified email validation
if (isValidEmail) {
console.log("Email is valid.");
// Proceed with form submission
} else {
console.log("Invalid email address.");
// Display an error message
}
In this example, the email
variable stores the user’s input, and the isValidEmail
variable stores the result of a basic validation check.
Best practices for using Variables
- Use descriptive names: Choose names that clearly indicate the variable’s purpose (e.g.,
userName
instead ofx
). - Follow naming conventions: Stick to camelCase (e.g.,
myVariableName
) for variable names. - Prefer
let
andconst
: Uselet
for variables that may be reassigned andconst
for constants. Avoidvar
unless you have a specific reason to use it. - Initialize variables when you declare them: This helps prevent undefined values and makes your code easier to read.
- Keep scope in mind: Be aware of the scope of your variables to avoid naming conflicts and unexpected behaviour.
Conclusion
Variables are the essential building blocks of any JavaScript program. By understanding how to declare, initialize, and use them effectively, you’ll be well on your way to mastering this powerful language. Remember to practice regularly and experiment with different data types and scopes to solidify your knowledge.
Happy coding!
Get up to 85% off
Build your project using Managed VPS & Dedicated hosting and decrease your workload.
You'll get seamless migration, exceptional savings, 24/7 technical support, HIPAA compliant, PCI compliant, Multi-Level DDoS protection, 30-day money-back guarantee and more with Liquid Web.
Affiliate Disclosure: Affiliate links are available here. If you click through the affiliate links and buy something, we may get a small commission, and you'll not be charged extra.